Follow Us:
How to Use Supabase Edge Functions for Low-Latency Applications?
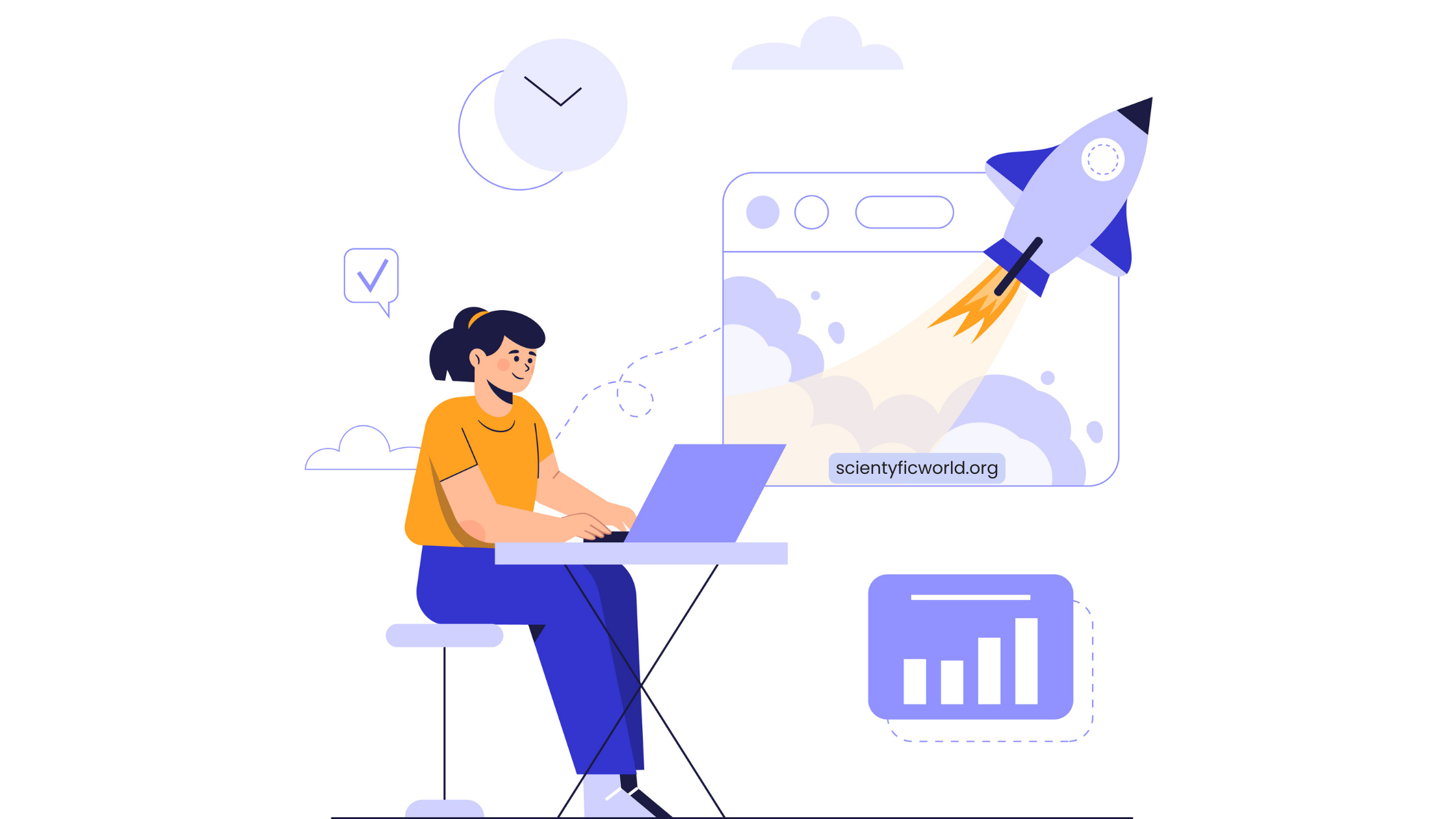
Welcome to a comprehensive guide on optimizing Supabase edge functions for applications demanding low-latency responses. In today’s fast-paced digital landscape, where immediacy is not just valued but expected, the efficiency of serverless functions becomes pivotal, particularly in fields like gaming and real-time analytics.
Supabase, a robust backend as a service, offers an enticing avenue for deploying serverless functions. These functions run close to the user, significantly reducing latency and enhancing user experience. This article targets intermediate and advanced developers, especially those working with React Native, who seek to refine their serverless solutions for speed and efficiency.
In this journey, we dive deep into the realms of serverless architecture within Supabase. We explore how to craft, deploy, and optimize serverless edge functions tailored for low latency. Whether you’re looking to boost your application’s real-time data processing or improve the responsiveness of your gaming app, this guide serves as your roadmap to achieving new heights in performance.
Understanding Supabase Edge Functions
Supabase Serverless Edge Functions are a powerful feature that allows developers to run backend code without managing a server infrastructure. These functions operate at the ‘edge’ of the network, meaning they’re executed as close as possible to the user, reducing latency significantly. This is particularly beneficial for applications requiring real-time interactions, such as live analytics in React Native apps.
Supabase Edge Functions are designed to enhance the performance and efficiency of web applications by executing code closer to the user. The primary purpose of these functions is to reduce latency, which is the time taken for a data packet to travel between the user and the server. This is particularly crucial in applications where real-time interactions and immediate responses are essential, such as in online gaming, live streaming, financial trading platforms, and real-time analytics in React Native apps.
How Do They Work?
- Triggering: Edge functions in Supabase are event-driven. They are triggered by specific events within your application, such as a database update, a user action, or an HTTP request.
- Execution Environment: When triggered, the function executes in an environment geographically closer to the user. This proximity reduces the time taken for the request to travel, thereby decreasing latency.
- Stateless Nature: Each execution is stateless. This means every execution is independent, and the function doesn’t retain any state between executions.
- Scalability: Supabase automatically scales these functions, managing the load without any manual intervention, ensuring consistent performance irrespective of the number of requests.
Workflow Overview:
The workflow of serverless edge functions in Supabase, especially for real-time analytics in a React Native application, is a multi-step process designed to maximize efficiency and minimize latency. Here’s a more detailed breakdown:
- User Interaction: It all starts with the user interacting with the React Native application. This could be any action like a click, view, or other engagement within the app.
- Triggering the Function: The app responds to this interaction by sending data to a specific Supabase serverless function. This function is configured to trigger upon receiving such data.
- Function Execution: Once triggered, the serverless function, running on an edge node close to the user, executes its code. This typically involves communicating with the Supabase database to query or update data based on the user’s action.
- Database Interaction: The function interacts with the Supabase database – querying, inserting, or updating records. The proximity of the database to the edge node enhances this interaction’s speed.
- Data Analysis: After database interaction, the function might send data to an analytics service for real-time analysis. This service processes the data, perhaps aggregating or analyzing user interactions, to derive meaningful insights.
- Processing and Response: The analytics service sends back processed data to the serverless function. The function then formulates a response, which could include analysis results or confirmation of a successful operation.
- Updating the User Interface: The serverless function sends this response back to the React Native app. The app then updates the user interface accordingly – displaying new content, analysis results, or confirmation messages.
- User Feedback: Finally, the user sees the results of their interaction, now enriched with real-time analysis or updates, completing the interaction loop.
This workflow exemplifies a seamless, efficient process where serverless edge functions significantly reduce response times, thereby enhancing the overall user experience in real-time applications.
Prerequisites
Before we delve into the optimization of serverless edge functions in Supabase, let’s establish the advanced prerequisites necessary for this endeavour. This section assumes you have a working knowledge of Supabase and a React Native environment setup. Our focus here is on the specific tools and configurations that will enable you to effectively integrate and optimize serverless functions in your React Native applications.
1. Supabase JavaScript Client Installation:
To interact with Supabase services, you need the Supabase JavaScript client. Install it using npm or yarn:
npm install @supabase/supabase-js
or,
yarn add @supabase/supabase-js
PowerShell2. Environment Configuration:
Set up your environment variables in your React Native project. Store your Supabase URL and Anon Key securely. You can use .env
files or a similar approach to manage these variables.
import { createClient } from '@supabase/supabase-js'
const SUPABASE_URL = 'your-supabase-url'
const SUPABASE_ANON_KEY = 'your-supabase-anon-key'
const supabase = createClient(SUPABASE_URL, SUPABASE_ANON_KEY)
JavaScript3. Advanced Tooling:
For debugging and monitoring performance, integrate advanced tooling into your development environment. Tools like React Native Debugger or Flipper can provide insights into performance bottlenecks and help optimize your serverless functions.
4. Understanding of Supabase Realtime and Functions:
A solid understanding of how Supabase’s real-time capabilities and serverless functions operate is crucial. Familiarize yourself with Supabase documentation on these topics to leverage their full potential.
5. Knowledge of Asynchronous JavaScript:
Since serverless functions often deal with asynchronous operations, a deep understanding of async-await patterns, Promises, and JavaScript’s event loop is essential.
By ensuring these prerequisites are in place, you equip yourself with the tools and knowledge needed to effectively optimize serverless edge functions in Supabase for low-latency applications. Next, we’ll explore the architecture of serverless functions in Supabase and how to design them for optimal performance.
Creating a Serverless Edge Function in React Native:
To create and use a serverless edge function in a React Native application, follow these steps:
- Define the Function: First, write the serverless function. This function should be designed to handle specific tasks like fetching, processing, and returning data.
// Example function to fetch user data
async function fetchUserData(userId) {
const { data, error } = await supabase
.from('users')
.select('*')
.eq('id', userId)
if (error) throw new Error(error.message);
return data;
}
JavaScript- Deploy the Function: Deploy this function to Supabase. You typically do this through the Supabase dashboard or CLI, where you define the event that triggers this function.
- Integrate with React Native: In your React Native application, make a call to this serverless function. This can be done through an HTTP request or using Supabase’s client library.
import { useEffect } from 'react';
const useUserData = (userId) => {
useEffect(() => {
const fetchData = async () => {
try {
const userData = await fetchUserData(userId);
console.log('User Data:', userData);
} catch (error) {
console.error('Error fetching user data:', error);
}
};
fetchData();
}, [userId]);
};
JavaScriptIn summary, Supabase Serverless Edge Functions provide a scalable, low-latency solution for React Native applications, especially useful for real-time data processing and analytics. By deploying code at the edge of the network, these functions offer a significant performance advantage, critical for applications where response time is crucial.
Designing Low-Latency Serverless Functions:
Designing serverless functions for low latency is crucial, especially for applications where response time is a key factor. The following steps outline how to design these functions efficiently within a React Native application using Supabase.
- Understand the Application Requirements:
Before writing any code, clearly define the requirements of your React Native application. Identify the operations that would benefit from low latency, such as real-time data fetching, processing, or updating. - Optimize Data Handling: Efficient data handling is essential. Structure your database queries to be as lean and efficient as possible. Avoid fetching unnecessary data.
Example:
// Efficient query to fetch only required data
async function fetchLatestMessages(chatId) {
const { data, error } = await supabase
.from('messages')
.select('id, content, timestamp')
.eq('chat_id', chatId)
.order('timestamp', { ascending: false })
.limit(50);
if (error) throw new Error(error.message);
return data;
}
JavaScript- Minimize External API Calls: If your function needs to call external APIs, ensure these calls are necessary. Cache responses where possible to avoid repeated calls.
- Use Asynchronous Programming Wisely: Leverage asynchronous programming to prevent blocking operations. Use async-await judiciously to handle concurrent operations effectively.
Example:
// Asynchronous function to handle multiple tasks
async function handleUserRequest(userId) {
try {
const [userData, userPreferences] = await Promise.all([
fetchUserData(userId),
fetchUserPreferences(userId)
]);
// Process and return combined data
return { userData, userPreferences };
} catch (error) {
console.error('Error in handling user request:', error);
}
}
JavaScript- Implement Efficient Error Handling: Efficient error handling ensures your function doesn’t fail silently and can recover or respond appropriately in case of issues.
- Keep Functions Lightweight: Each serverless function should be responsible for a single aspect of your application. Avoid creating large, monolithic functions. This separation enhances maintainability and can reduce latency, as smaller functions are quicker to deploy and execute.
- Regularly Monitor and Optimize Performance: Use tools like Supabase’s dashboard and logging to monitor the performance of your functions. Regularly review and optimize based on metrics and logs.
- Test Thoroughly: Thoroughly test each function in various scenarios to ensure they perform well under different loads. Automated testing can be beneficial here.
In React Native applications, designing low-latency serverless functions involves careful planning, efficient data handling, wise use of asynchronous programming, and regular performance monitoring. By following these steps, you can ensure that your serverless functions in Supabase are optimized for speed and efficiency, offering a seamless experience to the end users.
Implementing a Serverless Edge Function in Supabase:
Let’s dive into a practical implementation of a serverless edge function in Supabase, focusing on a real-time analytics use case for a React Native application. This example will illustrate how to capture and analyze user interactions in real time, providing valuable insights instantly.
Imagine a scenario where we want to track user interactions within our app – clicks, views, or other engagements – and analyze this data in real time. This analysis could help in understanding user behaviour, improving app performance, and enhancing user experience.
Step 1: Define the Serverless Function
First, we create a serverless function in Supabase that will be triggered every time a user interaction is recorded.
- Function Setup: In the Supabase dashboard, create a new serverless function. Let’s name it
trackUserInteraction
. - Function Code:
export default async function trackUserInteraction(req, res) {
const { userId, interactionType, timestamp } = req.body;
// Validate input
if (!userId || !interactionType || !timestamp) {
return res.status(400).json({ error: 'Missing required fields' });
}
try {
// Insert interaction data into the database
const { error } = await supabase
.from('interactions')
.insert([{ userId, interactionType, timestamp }]);
if (error) throw error;
// Optionally, perform real-time analysis here
// ...
res.status(200).json({ message: 'Interaction tracked successfully' });
} catch (error) {
res.status(500).json({ error: error.message });
}
}
JavaScriptStep 2: Integrate with React Native Application
Now, integrate this serverless function with the React Native application to send user interaction data.
- Interaction Tracking Function:
import { useEffect } from 'react';
const trackInteraction = async (userId, interactionType) => {
try {
const response = await fetch('supabase-function-url/trackUserInteraction', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
userId,
interactionType,
timestamp: new Date().toISOString(),
}),
});
const data = await response.json();
if (!response.ok) {
throw new Error(data.error);
}
} catch (error) {
console.error('Error tracking interaction:', error);
}
};
JavaScript- Usage Example:
const MyComponent = ({ userId }) => {
useEffect(() => {
// Example interaction tracking
trackInteraction(userId, 'viewed_my_component');
}, [userId]);
return (
// Component JSX
);
};
JavaScriptStep 3: Analyze the Data in Real-Time
With interactions being tracked, set up a real-time dashboard or analytics system within your app to display and analyze this data. You can use Supabase’s real-time subscriptions to listen for new interactions and update your analytics dashboard accordingly.
This implementation showcases how a serverless edge function in Supabase can efficiently handle real-time user interaction tracking in a React Native application. By leveraging the low-latency characteristics of serverless functions, we can gain instant insights into user behaviour, a crucial aspect of any dynamic application.
Optimizing Performance
Once we’ve implemented serverless edge functions in our Supabase and React Native setup, the next critical step is to optimize their performance. Effective optimization ensures that our low-latency functions run efficiently, providing the best possible experience to the end-users. Here’s how we can achieve this:
- Efficient Database Queries: Optimizing database queries is paramount. Ensure queries are concise and retrieve only the necessary data. Utilize indexing in your Supabase database to speed up query execution.
Example:
// Optimized query with specific fields and limit
const { data, error } = await supabase
.from('messages')
.select('id, content')
.eq('chat_id', chatId)
.order('timestamp', { ascending: false })
.limit(10);
JavaScript- Caching Strategies: Implement caching to reduce the number of times a function needs to fetch data. Use in-memory caching for frequently accessed data or implement a more robust caching mechanism like Redis if your application demands it.
- Code Optimization: Keep your serverless function code lean and efficient. Avoid unnecessary computations or heavy libraries that can slow down the execution.
- Parallel Processing: When dealing with multiple asynchronous operations, use
Promise.all
to handle them concurrently, reducing the overall execution time.
Example:
// Using Promise.all for concurrent operations
const [userData, userSettings] = await Promise.all([
fetchUserData(userId),
fetchUserSettings(userId)
]);
JavaScript- Function Warm-ups: To avoid cold starts (where the function takes longer to execute after a period of inactivity), implement a warm-up strategy. This can involve periodically triggering your functions to keep them ‘warm’.
- Performance Monitoring and Analysis: Regularly monitor your serverless functions using Supabase’s built-in analytics and logging. Identify any bottlenecks or performance issues and address them promptly.
- Optimize Network Calls: Minimize the size of payloads sent between your React Native app and the serverless functions. Use compression techniques if necessary to reduce the data transferred over the network.
- Environment Optimization: Ensure that your serverless environment is configured optimally. This includes setting appropriate memory and timeout settings for your functions based on their requirements.
Performance optimization is an ongoing process. By focusing on efficient database interactions, caching, code optimization, and regular monitoring, you can significantly enhance the speed and reliability of your serverless edge functions in Supabase, crucial for maintaining low latency in your React Native applications.
Handling Limitations and Challenges:
While serverless edge functions in Supabase offer a plethora of benefits, they also come with their own set of limitations and challenges. Understanding these and knowing how to navigate them is crucial for developers. Below is a table outlining common limitations and challenges along with strategies to handle them effectively.
Limitation/Challenge | Description | Handling Strategy |
---|---|---|
Cold Starts | A delay occurs when a serverless function is invoked after a period of inactivity, leading to a slower initial response. | Implement function warm-ups by periodically triggering functions. Optimize your code to reduce initialization time. |
Resource Limitations | Serverless functions have limits on memory, execution time, and concurrent executions. | Optimize function code to use resources efficiently. For memory-intensive tasks, consider splitting them into smaller, more manageable functions. Monitor and adjust function settings in Supabase as needed. |
State Management | Serverless functions are stateless, which can complicate scenarios requiring state persistence. | Utilize external storage solutions like Supabase database or caching mechanisms for state management. |
Debugging and Monitoring | Debugging serverless functions can be challenging due to their distributed nature and ephemeral execution environment. | Utilize logging extensively. Leverage Supabase’s function logs and monitoring tools. Employ local testing and third-party tools for a more thorough debugging process. |
Network Latency Variability | The latency can vary significantly based on the user’s location and the location of the nearest edge node. | Design your application to be resilient to variable network conditions. Use performance monitoring to understand and optimize for different scenarios. |
Security Concerns | Serverless functions, like any cloud resource, can be vulnerable to security threats. | Follow best practices for security, such as securing API endpoints, validating inputs, and using authentication and authorization mechanisms. Regularly update your functions to address any known vulnerabilities. |
Dependency Management | Managing dependencies in a serverless environment can be complex, especially when functions require external libraries. | Keep dependencies to a minimum. Use bundling tools to package only necessary parts of dependencies. Regularly update and audit dependencies for security and performance improvements. |
Testing Complexity | Testing serverless functions can be more complex due to the need to replicate the serverless environment and triggers. | Develop a comprehensive testing strategy that includes unit testing, integration testing, and end-to-end testing. Use emulators or local development environments to simulate the serverless environment. |
Vendor Lock-in | Using serverless functions from a specific provider like Supabase can lead to vendor lock-in, making it challenging to migrate to another service in the future. | Design your application with abstraction layers that allow for easier migration to different platforms. Keep business logic separate from vendor-specific code. |
This table provides a clear understanding of the various limitations and challenges associated with using Supabase serverless edge functions in a React Native application, along with strategies to effectively handle these issues. Being aware of these factors and proactively managing them ensures smoother development and deployment processes.
Conclusion
In this comprehensive guide, we’ve explored the intricacies of optimizing serverless edge functions in Supabase for low-latency applications, specifically tailored for React Native developers. From understanding the essence of Supabase serverless functions to implementing them in real-world scenarios like real-time analytics, we’ve covered a broad spectrum of essential aspects.
Key takeaways include the importance of designing efficient serverless functions, implementing best practices in data handling, and adopting strategies for performance optimization. We’ve also navigated through the common challenges and limitations, providing practical solutions to ensure robust and efficient application development.
Remember, the journey to optimize serverless functions is ongoing. Continuous learning, testing, and adapting to new challenges are part of the process. With this guide, you’re well-equipped to enhance your React Native applications with low-latency, efficient serverless functions in Supabase, paving the way for high-performance, scalable, and user-friendly applications.
This guide serves not just as a tutorial but as a gateway to innovative serverless solutions in the ever-evolving landscape of application development.