Follow Us:
How to build RESTful APIs with Node.js and Express?
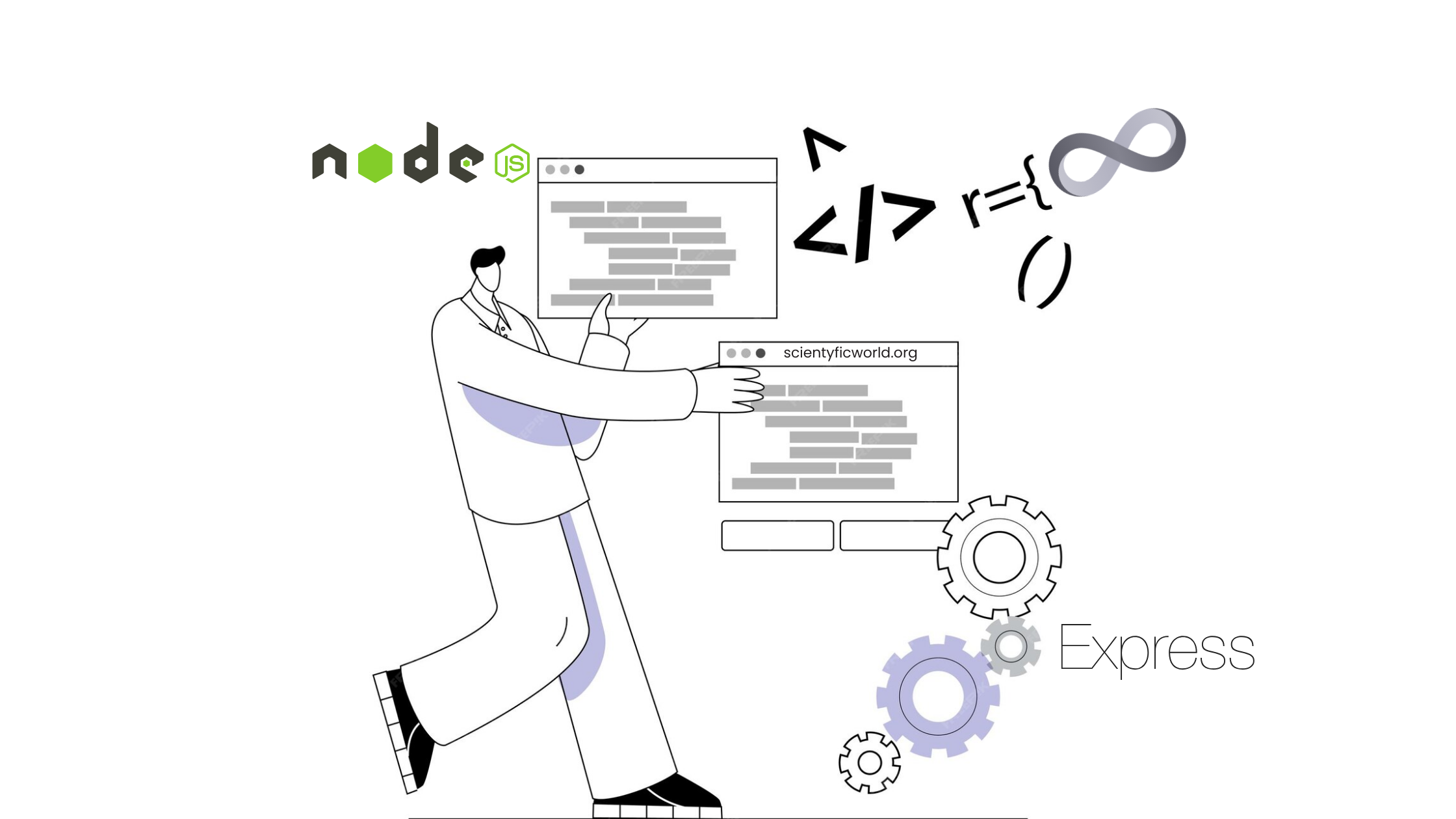
Welcome to our guide on building RESTful APIs with Node.js and Express. In this blog, we explore API development, guiding you through the process of creating, deploying, and managing a RESTful API.
RESTful APIs serve as a fundamental component in modern web services, enabling efficient communication between systems. They utilize HTTP protocols within a stateless, client-server architecture, crucial for scalable and flexible interactions across various applications and services.
Our emphasis is on Node.js and Express. Node.js is notable for its efficiency and scalability, while Express is a straightforward yet capable web application framework. Together, they form a suitable environment for developing effective APIs.
This guide is designed to be accessible to developers at all levels. Whether you’re a beginner, at an intermediate stage, or an experienced professional, this content is tailored for you. We present technical concepts in clear, direct language, focusing on active voice for better comprehension. Covering everything from setting up your development environment to diving into more advanced topics like security and deployment, our goal is to avoid redundancy and complexity in our explanations.
By the end of this blog, you will have a comprehensive understanding of RESTful APIs and be equipped with the skills to create one using Node.js and Express. Let’s begin this educational journey to enhance your set of development skills.
Setting Up the Development Environment
Before diving into the creation of a RESTful API, it’s crucial to set up a proper development environment. This foundation will ensure that you can smoothly develop, test, and deploy your application. Here’s how you can get started:
- Installing Node.js and npm
- Node.js: This is the runtime environment we’ll use to run our server-side JavaScript code. If you haven’t installed Node.js yet, download it from the official Node.js website. Choose the version recommended for most users.
- npm (Node Package Manager): It comes bundled with Node.js. npm is essential for managing packages that your project will depend on.
- Setting up a New Node.js Project
- Once Node.js and npm are installed, create a new directory for your project. You can do this using your operating system’s file explorer or via the command line.
- Open a terminal or command prompt, navigate to your project directory, and run
npm init
. This command starts the process of creating apackage.json
file for your project. You can fill in the details as prompted or press enter to accept default values.
- Understanding Basic Project Structure
- Package.json: This file holds various metadata relevant to the project. It is used to give information to npm that allows it to identify the project and handle its dependencies.
- Node_modules: When you install npm packages, they will be placed in a folder named
node_modules
. This folder is auto-generated and should not be modified manually. - Entry Point: Usually, an
index.js
orapp.js
file acts as the entry point of your Node.js application. This is where you will set up your Express server.
- Installing Express
- In your project directory, run
npm install express
. This command installs Express and adds it as a dependency in yourpackage.json
file. - After installation, you will see Express listed under the dependencies in
package.json
.
- In your project directory, run
- Version Control with Git (Optional but Recommended)
- Initialize a Git repository in your project folder by running
git init
. This step is optional but highly recommended for version control. - Create a
.gitignore
file to exclude thenode_modules
directory and other non-essential files from your Git repository.
- Initialize a Git repository in your project folder by running
With these steps, your development environment is now set up. This setup provides the foundation upon which we will build our RESTful API using Node.js and Express. In the next section, we will dive into understanding RESTful principles and how they apply to our project.
Understanding RESTful Principles
Before we start coding, it’s important to understand the principles of RESTful architecture.
A REST API (Representational State Transfer API) is an API that adheres to the principles of REST, a set of architectural guidelines for networked applications. REST APIs use HTTP requests to access and use data. That data can be used to GET, PUT, POST, and DELETE data types, which refers to the reading, updating, creating, and deleting of operations concerning resources.
Let’s break down these principles to understand how they apply to API development:
- Statelessness
- In REST, each HTTP request from a client to a server must contain all the information the server needs to execute the request. The server does not store any state about the client session on the server side. This makes the API scalable as the server does not need to maintain, manage, or communicate the session state.
- Client-Server Architecture
- This principle separates the user interface concerns from the data storage concerns, improving the portability of the user interface across multiple platforms and scalability by simplifying the server components.
- Uniform Interface
- The uniform interface simplifies the architecture by decoupling the client and server. It’s centred around four main principles:
- Resource Identification in Requests: Resources are identified in requests using URIs, which are typically links on the web.
- Resource Manipulation through Representations: When a client holds a representation of a resource, including any metadata attached, it has enough information to modify or delete the resource on the server, provided it has permission to do so.
- Self-descriptive Messages: Each message includes enough information to describe how to process it, which can be achieved using standard HTTP headers and status codes.
- Hypermedia as the Engine of Application State (HATEOAS): The client can discover all the actions it can perform on the server by examining the hypermedia links.
- The uniform interface simplifies the architecture by decoupling the client and server. It’s centred around four main principles:
- HTTP Methods
- REST APIs primarily use four HTTP methods:
- GET to retrieve a resource;
- POST to create a new resource;
- PUT to update an existing resource;
- DELETE to remove a resource.
- These methods correspond to the CRUD operations in databases.
- REST APIs primarily use four HTTP methods:
- Stateless Responses
- In line with being stateless, responses from the server to the client must also be self-contained. Responses should carry enough information for the client to process it without depending on the server’s state.
- Cacheability
- Responses must be defined as cacheable or non-cacheable. If a response is cacheable, the client can reuse the response data for later, equivalent responses, reducing the number of interactions needed between the client and the server.
Understanding these principles is crucial for building effective and efficient RESTful APIs. They ensure that the API is scalable, maintainable, and performant. In the next sections, we’ll see how these principles are applied in practice when building an API using Node.js and Express.
Getting Started with Express
Having understood the core principles of RESTful APIs, let’s move on to the practical aspect of building one using Express, a popular web application framework for Node.js. Express simplifies the development of web applications and APIs with its powerful features and straightforward approach.
Express is a minimal, flexible Node.js web application framework that provides a robust set of features to develop web and mobile applications. It makes it easy to create a server and routes, handle requests and responses, and integrate with databases.
Setting Up an Express Server
- Install Express: Assuming you have created your Node.js project and have a
package.json
file, you can install Express by running the commandnpm install express
in your project directory. - Create Your Main File: In your project directory, create a file named
app.js
(orindex.js
, depending on your preference). This file will serve as the entry point for your Express application. - Import Express and Set Up the Server: In
app.js
, start by importing the Express module and create an instance of an Express application. Set up the server to listen on a specific port. Here’s a basic setup:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
JavaScriptThis code, app.get
defines a route to handle GET requests to the root URL ('/'
). When this route is accessed, it responds with ‘Hello World!’.
Handling Routing with Express
Routing refers to how an application responds to a client request to a particular endpoint, which is a URI (or path) and a specific HTTP request method (GET, POST, etc.).
- Basic Routing: Express provides methods that correspond to HTTP request methods. For example,
app.get()
to handle GET requests,app.post()
for POST requests, etc. Here’s an example of a simple route:
app.get('/api/items', (req, res) => {
res.send('List of items');
});
JavaScriptThis route responds with ‘List of items’ when a GET request is made to /api/items
.
- Route Parameters: Express allows you to define routes with route parameters, making it easy to capture values specified at their position in the URL. For instance:
app.get('/api/items/:id', (req, res) => {
res.send(`Item ID: ${req.params.id}`);
});
JavaScriptHere, :id
is a route parameter that you can access via req.params.id
.
- Middleware: Express allows you to use middleware functions that have access to the request object (
req
), the response object (res
), and the next middleware function in the application’s request-response cycle. Middleware functions can execute code, make changes to the request and response objects, end the request-response cycle, or call the next middleware function.
app.use(express.json());
JavaScriptThis line uses the express.json()
middleware, which parses incoming requests with JSON payloads.
With your Express server set-up and a basic understanding of routing and middleware, you’re well on your way to building a fully functional RESTful API. In the following sections, we’ll explore how to build out the API with CRUD operations, connect to a database, handle errors, and more.
Building a Simple RESTful API
Now that we have our Express server up and running, let’s dive into building a simple RESTful API. In this section, we will create an API that performs basic CRUD (Create, Read, Update, Delete) operations. We’ll start with a simple in-memory data structure, which we will later replace with a database connection.
Step 1: Define the Data Structure
For simplicity, let’s assume we are building an API for managing a list of tasks. We will use an array of objects, each representing a task:
let tasks = [
{ id: 1, title: "Task 1", completed: false },
{ id: 2, title: "Task 2", completed: false },
// more tasks
];
JavaScriptStep 2: Implementing CRUD Operations
- Create (POST) a New Task
- To add a new task, we’ll use a POST request.
- Ensure your Express app can handle JSON requests by using
app.use(express.json())
. - Define a POST route to add a new task.
app.post('/api/tasks', (req, res) => {
const task = {
id: tasks.length + 1,
title: req.body.title,
completed: false
};
tasks.push(task);
res.send(task);
});
JavaScript- Read (GET) Tasks
- To retrieve all tasks or a single task, we’ll use GET requests.
- Define GET routes for fetching all tasks and a specific task by ID.
app.get('/api/tasks', (req, res) => {
res.send(tasks);
});
app.get('/api/tasks/:id', (req, res) => {
const task = tasks.find(t => t.id === parseInt(req.params.id));
if (!task) return res.status(404).send('Task not found.');
res.send(task);
});
JavaScript- Update (PUT) a Task
- To update a task, we’ll use a PUT request.
- Define a PUT route for updating a specific task.
app.put('/api/tasks/:id', (req, res) => {
const task = tasks.find(t => t.id === parseInt(req.params.id));
if (!task) return res.status(404).send('Task not found.');
task.title = req.body.title;
task.completed = req.body.completed;
res.send(task);
});
JavaScript- Delete (DELETE) a Task
- To delete a task, we’ll use a DELETE request.
- Define a DELETE route for removing a specific task.
app.delete('/api/tasks/:id', (req, res) => {
const task = tasks.find(t => t.id === parseInt(req.params.id));
if (!task) return res.status(404).send('Task not found.');
const index = tasks.indexOf(task);
tasks.splice(index, 1);
res.send(task);
});
JavaScriptStep 3: Testing Your API
You can test your API using tools like Postman or cURL. Make requests to your API endpoints and verify if the operations (create, read, update, delete) are working as expected.
You now have a basic RESTful API for managing tasks, with CRUD operations implemented. This API currently stores data in memory, which means data will be lost when the server restarts. In the following sections, we will enhance this API by connecting it to a database, adding error handling, and exploring more advanced features.
Working with Middleware in Express
Middleware functions are an integral part of developing applications with Express. They can execute code, make changes to the request and response objects, end the request-response cycle, or call the next middleware in the stack. Understanding how to effectively use middleware is key to building efficient and secure Express applications.
Middleware functions have access to the request object (
req
), the response object (res
), and the next function in the application’s request-response cycle. This “next” function is a function in the Express router which, when invoked, executes the middleware succeeding the current middleware.
Types of Middleware
- Application-level Middleware
- These are bound to an instance of Express, using
app.use()
andapp.METHOD()
, where METHOD is the HTTP method (like GET, PUT). - They can be used for logging, user authentication, etc.
- These are bound to an instance of Express, using
- Router-level Middleware
- They work in the same way as application-level middleware but are bound to an instance of
express.Router()
.
- They work in the same way as application-level middleware but are bound to an instance of
- Error-handling Middleware
- Defined using four arguments instead of three, with the first one being an error.
- Built-in Middleware
- Express comes with built-in middleware functions, such as
express.static
(to serve static files) andexpress.json
(to parse JSON requests).
- Express comes with built-in middleware functions, such as
- Third-party Middleware
- You can install additional middleware modules like
body-parser
,morgan
, orcors
to extend the functionality of your Express app.
- You can install additional middleware modules like
Using Middleware
- Global Middleware
- To use middleware across all routes in the app, use
app.use()
. - For example, to parse JSON bodies of requests, use:
app.use(express.json());
- To use middleware across all routes in the app, use
- Route-specific Middleware
- To apply middleware to a specific route, pass the middleware function as an argument to the route.
const log = (req, res, next) => {
console.log('Logging...');
next();
};
app.get('/api/tasks', log, (req, res) => {
// Your route handler
});
JavaScript3. Error Handling
- Write error-handling middleware to handle errors uniformly across the application.
- This middleware should be defined last, after all other
app.use()
and route calls.
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
JavaScriptLet’s create a simple logging middleware that prints the request method and URL every time a request is made:
const logger = (req, res, next) => {
console.log(`${req.method} ${req.url}`);
next();
};
app.use(logger);
JavaScriptBy placing app.use(logger)
at the top of your middleware stack (just after initializing your Express app), every request to your server will pass through this logger middleware.
Middleware functions are powerful tools for managing the request-response cycle, handling errors, and extending your application’s capabilities. By understanding and effectively utilizing middleware, you can significantly enhance the functionality and reliability of your Express applications.
Data Handling and Persistence
In the previous sections, we developed a RESTful API with in-memory data storage. While this is useful for demonstration purposes, most applications require persistent data storage, typically using a database. This section will guide you through integrating a database with your Express application for robust data handling and persistence.
Choosing a Database
- SQL vs NoSQL Databases
- SQL Databases (like MySQL, PostgreSQL): They use structured query language (SQL) for defining and manipulating data. They are a good choice for applications with complex queries and transactions.
- NoSQL Databases (like MongoDB, Firebase or Supabase): They are more flexible in terms of data models and are suitable for large data sets and real-time applications.
- Factors to Consider
- Data Structure: Whether your data is structured, semi-structured, or unstructured.
- Scalability: How the database scales, vertically or horizontally.
- Consistency: The level of consistency required by your application.
Setting Up a Database
For demonstration, let’s assume we are using MongoDB, a popular NoSQL database. To integrate MongoDB with our Express application:
- Install MongoDB: Follow the official MongoDB installation guide for your operating system.
- Install Mongoose: Mongoose is an ODM (Object Data Modeling) library for MongoDB and Node.js. It manages relationships between data, provides schema validation, and translates between objects in code and the representation of those objects in MongoDB.
npm install mongoose
JavaScript- Connecting to MongoDB
- In your main application file, import Mongoose and connect to your MongoDB database.
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/yourdbname', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB...'))
.catch(err => console.error('Could not connect to MongoDB...', err));
JavaScriptDefining a Model
- Create a Schema: Define a schema that represents a task, using Mongoose’s schema interface.
const taskSchema = new mongoose.Schema({
title: String,
completed: Boolean,
dateCreated: { type: Date, default: Date.now }
});
JavaScript- Create a Model: Compile the schema into a model. A model is a class with which you construct documents.
const Task = mongoose.model('Task', taskSchema);
JavaScriptImplementing CRUD Operations with the Database
- Create: Insert a new task into the database.
app.post('/api/tasks', async (req, res) => {
let task = new Task({
title: req.body.title,
completed: req.body.completed
});
task = await task.save();
res.send(task);
});
JavaScript- Read: Fetch tasks from the database.
app.get('/api/tasks', async (req, res) => {
const tasks = await Task.find();
res.send(tasks);
});
JavaScript- Update: Update an existing task.
app.put('/api/tasks/:id', async (req, res) => {
const task = await Task.findByIdAndUpdate(req.params.id, {
title: req.body.title,
completed: req.body.completed
}, { new: true });
if (!task) return res.status(404).send('The task with the given ID was not found.');
res.send(task);
});
JavaScript- Delete: Delete a task.
app.delete('/api/tasks/:id', async (req, res) => {
const task = await Task.findByIdAndRemove(req.params.id);
if (!task) return res.status(404).send('The task with the given ID was not found.');
res.send(task);
});
JavaScriptIntegrating a database into your Express application allows for persistent data storage, making your application more robust and scalable. While this example uses MongoDB, the principles apply to other databases as well. Proper database integration is crucial for handling and persisting data effectively in your RESTful API.
Error Handling and Validation
A critical aspect of any robust RESTful API is effective error handling and validation. Proper error handling ensures that your API responds with the appropriate status codes and messages when something goes wrong. Validation, on the other hand, ensures that the data provided by the client meets certain criteria before it’s processed by the server.
Error Handling in Express
- Basic Error Handling
- In Express, you can define error-handling middleware functions that have four arguments instead of three:
(err, req, res, next)
. This middleware is used to catch and respond to errors in your application. - Place your error-handling middleware at the end of all other middleware and route handlers:
- In Express, you can define error-handling middleware functions that have four arguments instead of three:
app.use((err, req, res, next) => {
console.error(err.message);
res.status(500).send('Something failed.');
});
JavaScript- Handling Asynchronous Errors
- Asynchronous functions in Express do not pass errors to your error-handling middleware automatically. You need to catch these errors and pass them to the next function manually.
- Use
try...catch
blocks or promise chaining to handle errors in asynchronous operations.
- Custom Error Classes
- For better control and readability, consider creating custom error classes.
- These classes can encapsulate specific error messages and status codes.
Data Validation
- Why Validate?
- Validation is crucial to protect your API from invalid or malicious data.
- It ensures that the data fits your application’s requirements before it gets processed.
- Implementing Validation
- You can implement validation manually in your route handlers, or use a library like Joi.
- Joi is a powerful schema description language and data validator for JavaScript.
- Example Using Joi
- First, install Joi:
npm install joi
. - Define a schema corresponding to your data structure and use it to validate request bodies.
- First, install Joi:
const Joi = require('joi');
const taskSchema = Joi.object({
title: Joi.string().min(3).required(),
completed: Joi.boolean()
});
app.post('/api/tasks', async (req, res) => {
const { error } = taskSchema.validate(req.body);
if (error) return res.status(400).send(error.details[0].message);
// Rest of the code to create a task
});
JavaScriptCombined Error Handling and Validation
- Centralizing Error Handling
- Create a middleware function for validation and use it across different routes.
- Handle validation errors in the same place where you handle other types of errors for consistency.
- Error Response Structure: Provide a consistent structure for error responses, including an error code, message, and possibly other details.
- Logging: Log errors for diagnostic purposes. Consider using a logging library for more advanced logging requirements.
Effective error handling and validation are key to the stability and security of your RESTful API. They not only prevent unexpected crashes and data corruption but also provide a clear and informative feedback mechanism for the clients interacting with your API. Implementing these practices will significantly enhance the reliability and usability of your application.
Securing the API
Securing your RESTful API is crucial to protect sensitive data and ensure that only authorized users can access certain functionalities. In this section, we’ll cover essential security practices for your Node.js and Express application.
Authentication and Authorization
- Authentication
- Authentication verifies who the user is. This typically involves a username and password, but can also include other methods like tokens or biometric data.
- Implementing Authentication:
- Use packages like
passport
orjsonwebtoken
for implementing authentication. - For token-based authentication, JSON Web Tokens (JWT) are widely used. After a user logs in, the server creates a token and sends it back to the client. This token is then used for subsequent requests to the server.
- Use packages like
- Authorization
- Authorization determines what an authenticated user is allowed to do.
- Implement access control in your routes to ensure users can only access the resources they are permitted to.
Using JSON Web Tokens (JWT)
- Setting Up JWT
- Install the necessary package:
npm install jsonwebtoken
.When a user logs in, generate a JWT and send it back to the client.
- Install the necessary package:
const jwt = require('jsonwebtoken');
app.post('/api/login', (req, res) => {
// Validate user credentials...
const token = jwt.sign({ userId: user._id }, 'yourPrivateKey');
res.send({ token });
});
JavaScript- Verifying JWT
- Create middleware to verify the token on protected routes.The middleware checks if the request has a valid JWT before allowing access to the route.
function authenticateToken(req, res, next) {
const token = req.header('Authorization');
if (!token) return res.status(401).send('Access denied. No token provided.');
try {
const decoded = jwt.verify(token, 'yourPrivateKey');
req.user = decoded;
next();
} catch (ex) {
res.status(400).send('Invalid token.');
}
}
JavaScriptOther Security Best Practices
- HTTPS
- Use HTTPS to encrypt data in transit. This is critical for protecting sensitive data like passwords and personal information.
- For local development, you can use tools like
ngrok
to tunnel your local server. For production, ensure your hosting provider supports HTTPS.
- Helmet
- Helmet is a middleware for Express that sets various HTTP headers to secure your app.
- Install it using
npm install helmet
and use it withapp.use(helmet())
.
- Rate Limiting
- Protect against brute-force attacks by limiting the number of requests a user can make in a given period.
- Implement rate limiting using libraries like
express-rate-limit
.
- Cross-Origin Resource Sharing (CORS)
- If your API is accessed from different domains, you need to enable CORS.
- Use the
cors
middleware for Express (npm install cors
).
- Input Validation
- Always validate and sanitize user input to protect against SQL injection, XSS, and other attacks.
- Use libraries like Joi or express-validator for input validation.
- Dependency Management
- Regularly update your dependencies to include security patches.
- Use tools like
npm audit
to identify and fix security vulnerabilities.
Securing your API is an ongoing process that involves multiple layers of protection. From authenticating users to encrypting data and managing cross-origin requests, each aspect plays a vital role in safeguarding the data and integrity of your application. Implementing these security measures will help you build a reliable and secure RESTful API with Node.js and Express.
Testing the API
Testing is a critical part of API development, ensuring that your application behaves as expected and helping to catch bugs and issues early in the development cycle. This section will cover the key aspects of testing your RESTful API built with Node.js and Express.
Types of Tests
- Unit Tests
- Focus on testing individual parts of the code in isolation (e.g., functions, middleware).
- Useful for ensuring that each component of your API works correctly on its own.
- Integration Tests
- Test the interactions between various parts of the application, including external services like databases.
- Particularly important for testing your endpoints and the interaction with the database.
- End-to-End (E2E) Tests
- Simulate real user scenarios from start to finish.
- Useful for testing the entire application flow.
Setting Up the Testing Environment
- Choose a Testing Framework
- Popular frameworks for Node.js include Mocha, Jest, and Jasmine.
- Mocha is widely used for Node.js applications and pairs well with assertion libraries like Chai.
- Installing Mocha and Chai
- Install Mocha and Chai as development dependencies:
npm install --save-dev mocha chai
- Install Mocha and Chai as development dependencies:
- Configure a Test Script
- In your
package.json
, add a script to run your tests:
- In your
"scripts": {
"test": "mocha"
}
JavaScriptWriting Tests
- Unit Testing
- Write tests for individual functions and middleware.
- Use
describe
to group tests andit
for individual test cases. - Use Chai’s expect or assert functions to test conditions.
describe('Task Operations', () => {
it('should create a new task', () => {
let task = { title: 'Test Task' };
// Logic to add the task
expect(task).to.have.property('id');
});
// Additional tests...
});
JavaScript- Integration Testing
- Test the API endpoints.Use Chai HTTP for making HTTP requests and asserting the response.
const chaiHttp = require('chai-http');
const app = require('../app');
chai.use(chaiHttp);
describe('API Routes', () => {
it('GET /api/tasks should return all tasks', done => {
chai.request(app)
.get('/api/tasks')
.end((err, res) => {
expect(res).to.have.status(200);
expect(res.body).to.be.an('array');
done();
});
});
// Additional endpoint tests...
});
JavaScriptMocking and Stubbing
- Using Mocks and Stubs
- For components like databases or external services, use mocking and stubbing to simulate their behaviour.
- Libraries like Sinon can be used for this purpose.
- Mocking External Services
- Replace real HTTP requests with mock responses.
- This ensures your tests are not reliant on external services and are more reliable and faster.
Continuous Integration (CI)
- Implement CI Tools
- Use CI/CD tools like Jenkins, Travis CI, or GitHub Actions to automate your testing process.
- CI tools can automatically run your tests on different environments and notify you of failures.
Thorough testing is essential for building reliable and robust RESTful APIs. By incorporating unit, integration, and E2E tests, you can ensure that each component of your application functions correctly both individually and as part of the whole. Regular and automated testing not only helps in identifying bugs early but also aids in maintaining the overall quality of your application as it evolves.
Deploying the API
Deploying your RESTful API is the final step in making it accessible to users. This section provides a brief overview of the key steps involved in deploying a Node.js and Express application.
Choosing a Hosting Provider
- Options for Deployment
- There are several cloud-based hosting providers like Heroku, AWS (Amazon Web Services), Azure, and Google Cloud Platform. These services offer varying degrees of control, scalability, and ease of use.
- Heroku for Simplicity
- Heroku is a popular choice for its simplicity and ease of use, especially for small to medium-sized projects. It offers a straightforward deployment process and a free tier for small projects.
Preparing for Deployment
- Environment Variables
- Move configuration settings like database URIs, secret keys, and API keys to environment variables for security and flexibility.
- Use libraries like
dotenv
to manage environment variables in your development environment.
- Production Database
- Set up a production-ready database. Cloud providers often offer their database services, or you can use third-party services.
- Optimizations
- Ensure your code is optimized for production. This includes minifying code, optimizing performance, and ensuring efficient database queries.
Deployment Process
- Heroku Deployment Example
- Create a Heroku account and install the Heroku CLI.
- Log in to Heroku through the CLI and create a new Heroku app.
- Initialize a Git repository in your project directory if you haven’t already.
- Connect your Git repository to Heroku using
heroku git:remote -a your-app-name
. - Deploy your application using Git:
git push heroku master
. - Your application should now be live on Heroku.
- Post-Deployment Steps
- After deployment, monitor your application for any issues.
- Set up logging and error monitoring services (like New Relic or Sentry) to keep track of your application’s health and performance.
Deploying your RESTful API makes it accessible over the internet, allowing users to interact with it. While the process may vary depending on the hosting provider and the specific requirements of your application, the basic principles of preparing your application for the production environment and deploying it through a hosting service remain consistent. Regular monitoring and maintenance post-deployment are crucial for ensuring the long-term success and reliability of your API.
Conclusion
In this comprehensive guide, we’ve journeyed through the process of building a RESTful API using Node.js and Express. Starting from setting up the development environment, we covered the fundamentals of RESTful principles, implemented CRUD operations, utilized middleware for enhanced functionality, and integrated a database for persistent data storage.
Key aspects like error handling, validation, and security were addressed to ensure that the API is robust and reliable. We also touched on the importance of testing in maintaining the quality of the API and concluded with deployment strategies to make the API accessible to users.
Remember, building an API is not just about getting it to work; it’s about creating a solution that is efficient, secure, and easy to maintain. The topics covered here provide a solid foundation, but continuous learning and adapting to new challenges are part of a developer’s journey.
As you move forward, experiment with different tools and technologies, and always keep best practices in mind to refine and enhance your skills in API development.