Follow Us:
Protocols in Socket Programming
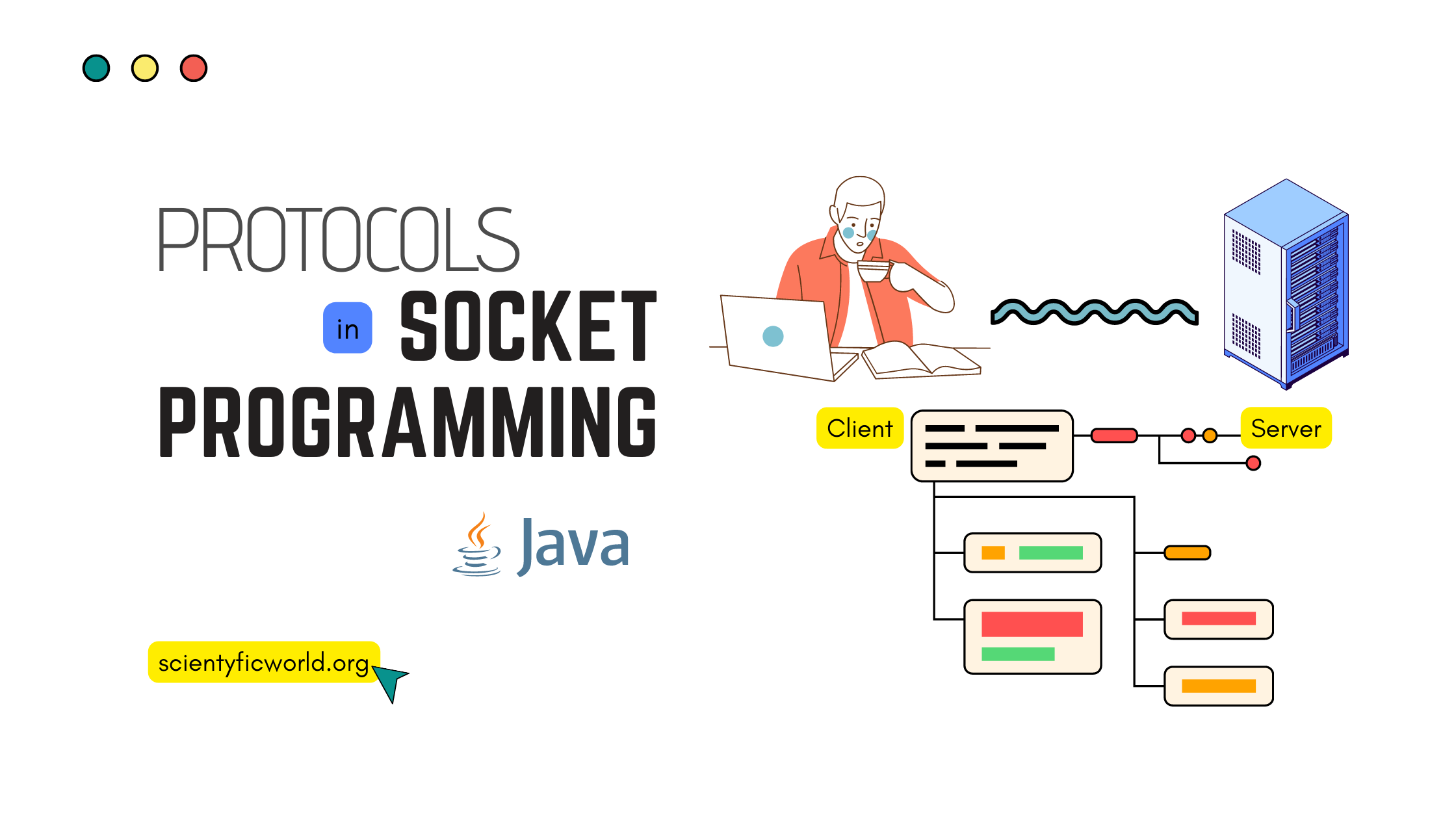
In our previous blog, “Socket Programming in Java,” we explored the fundamentals of establishing connections and exchanging data. Now, it’s time to delve deeper into the realm of protocols in socket programming.
Protocols play a vital role in ensuring reliable data transmission between communicating entities. They tackle challenges such as data loss, errors, and congestion, guaranteeing the integrity and efficiency of the transmitted information. In this blog, we will focus on two main protocols: the Stop and Wait protocol and the Sliding Window protocol, both widely used in socket programming.
We will also discuss the Automatic Repeat Request (ARQ) mechanism associated with each protocol. ARQ enables the detection and retransmission of lost or corrupted data, further enhancing the reliability of communication.
Throughout this guide, we will provide you with a comprehensive understanding of these protocols, their ARQ strategies, and how to implement them in Java. So, let’s dive in and explore the fascinating world of protocols in socket programming.
Understanding Protocols in Socket Programming
Protocols, in the context of socket programming, are a set of rules and guidelines that govern the communication between a client and a server over a network. These protocols define the format, order, and semantics of the messages exchanged, ensuring that both ends can interpret and process the data correctly.
Protocols establish a common language for communication, enabling different software applications to interact seamlessly. They define how data is structured, how errors are handled, and how reliable delivery is achieved. Without protocols, the chaos would reign, and reliable communication would be nearly impossible.
Importance of Protocols in Socket Programming:
In socket programming, protocols play a crucial role in facilitating reliable and efficient data transmission between client and server. Here are a few key reasons why protocols are essential:
- Standardization: Protocols provide a standardized framework for communication, ensuring that various software applications can interoperate regardless of their implementation details.
- Data Integrity: Protocols incorporate mechanisms to verify the integrity of the transmitted data, such as error detection codes and checksums. These mechanisms help detect and correct errors that may occur during transmission.
- Flow Control: Protocols manage the flow of data between the sender and receiver, preventing data overload or congestion. They ensure that the receiving end can handle the incoming data at a pace it can process.
- Error Recovery: Protocols include error recovery mechanisms to handle lost, duplicated, or corrupted data. These mechanisms, such as the Automatic Repeat Request (ARQ), enable the retransmission of faulty data to ensure its reliable delivery.
- Efficiency: By optimizing data transmission, protocols enhance the overall efficiency of communication. They minimize overhead, reduce latency, and maximize the utilization of network resources.
Understanding protocols is fundamental to building robust and reliable network applications. In the following sections, we will explore two main protocols in socket programming: the Stop and Wait protocol and the Sliding Window protocol. We will also dive into their respective ARQ mechanisms and learn how to implement them in Java.
Stop and Wait Protocol:
The Stop and Wait protocol is a simple and straightforward protocol used for reliable data transmission between a sender and a receiver in socket programming. In this protocol, the sender sends a data packet to the receiver and waits for an acknowledgment (ACK) before sending the next packet. The receiver, upon receiving the packet, sends an acknowledgment back to the sender indicating successful reception.
Implementing Stop and Wait Protocol in Java:
To understand the Stop and Wait protocol, let’s delve into the detailed steps involved and how it works:
- Sender’s Perspective:
- The sender prepares a data packet to be transmitted to the receiver.
- The sender sends the data packet to the receiver.
- The sender starts a timer and waits for an acknowledgment (ACK) from the receiver.
- Receiver’s Perspective:
- The receiver awaits the arrival of a data packet from the sender.
- Upon receiving the data packet, the receiver sends an acknowledgment (ACK) back to the sender.
- The receiver processes the received data packet.
- Sender’s Handling of ACK:
- If the sender receives the ACK within the specified timeout period:
- The sender stops the timer.
- The sender proceeds to send the next data packet.
- If the sender does not receive the ACK within the timeout period:
- The sender assumes that the data packet or the ACK is lost or corrupted.
- The sender retransmits the same data packet.
- The sender restarts the timer and waits for the ACK.
- If the sender receives the ACK within the specified timeout period:
- Receiver’s Handling of Data Packet:
- If the receiver receives a valid data packet:
- The receiver sends an ACK back to the sender to acknowledge successful receipt.
- The receiver processes the data packet and extracts the relevant information.
- If the receiver receives a duplicate or corrupted data packet:
- The receiver ignores the duplicate packet and does not send an ACK.
- The receiver may also send a negative acknowledgment (NAK) to inform the sender of the error.
- If the receiver receives a valid data packet:
This process continues until all the data packets are successfully transmitted and acknowledged.
Server program:
import java.io.*;
import java.net.*;
public class Server {
public static void main(String[] args) {
try {
ServerSocket serverSocket = new ServerSocket(8000);
System.out.println("Server started. Waiting for a connection...");
Socket socket = serverSocket.accept();
System.out.println("Client connected.");
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
String data = in.readLine();
System.out.println("Received data: " + data);
// Process the received data
out.println("ACK");
socket.close();
serverSocket.close();
System.out.println("Connection closed.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
JavaClient program:
import java.io.*;
import java.net.*;
public class Client {
public static void main(String[] args) {
try {
Socket socket = new Socket("localhost", 8000);
System.out.println("Connected to server.");
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
String data = "Hello, Server!";
out.println(data);
System.out.println("Sent data: " + data);
String ack = in.readLine();
System.out.println("Received ACK: " + ack);
socket.close();
System.out.println("Connection closed.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
JavaAdvantages:
- Simplicity: The Stop and Wait protocol is easy to understand and implement.
- Flow Control: It provides a basic form of flow control by ensuring that the sender does not overwhelm the receiver with data.
Drawbacks:
- Low Efficiency: The Stop and Wait protocol has low efficiency due to the sender waiting for an acknowledgment before sending the next packet. This introduces significant delays, especially in high-latency networks.
- Limited Throughput: The protocol does not allow for pipelining or concurrent transmission, resulting in lower throughput compared to other protocols.
- Increased Overhead: The need for acknowledgments for every packet sent increases the overhead of the communication.
The Stop and Wait protocol ensures reliability by requiring the sender to receive an acknowledgment for each transmitted data packet before sending the next one. This allows the sender to retransmit any lost or corrupted packets. However, this simplicity comes at the cost of reduced efficiency, as the sender has to wait for the acknowledgment before proceeding. The protocol is more suitable for scenarios with low latency and minimal data loss.
Stop-and-Wait Automatic Repeat Request (ARQ) was introduced to improve the problem of unreliable data transmission and ensure reliable delivery by implementing a mechanism for error detection and retransmission in the Stop and Wait protocol.
Stop-and-Wait Automatic Repeat Request (ARQ)
The Stop-and-Wait Automatic Repeat Request (ARQ) protocol is an enhanced version of the basic Stop and Wait protocol. It addresses the issue of unreliable data transmission by introducing error detection and retransmission mechanisms. This protocol ensures that data packets are successfully delivered and received by implementing acknowledgments and timeouts.
The Stop-and-Wait Automatic Repeat Request (ARQ) protocol is an enhanced version of the basic Stop and Wait protocol that adds error detection and retransmission mechanisms to ensure reliable data transmission between a sender and a receiver. Let’s dive into the steps involved and how the protocol works:
- Sender’s Perspective:
- The sender prepares a data packet to be transmitted to the receiver.
- The sender sends the data packet to the receiver.
- The sender starts a timer and waits for an acknowledgment (ACK) or negative acknowledgment (NAK) from the receiver.
- Receiver’s Perspective:
- The receiver awaits the arrival of a data packet from the sender.
- Upon receiving the data packet, the receiver performs error detection checks to ensure the integrity of the packet.
- If the data packet is error-free, the receiver sends an ACK back to the sender.
- If the data packet is corrupted or lost, the receiver sends a NAK back to the sender.
- Sender’s Handling of ACK and NAK:
- If the sender receives an ACK within the specified timeout period:
- The sender stops the timer.
- The sender proceeds to send the next data packet.
- If the sender receives a NAK within the timeout period:
- The sender assumes that the data packet was corrupted or lost.
- The sender retransmits the same data packet.
- The sender restarts the timer and waits for the ACK/NAK response.
- If the sender receives an ACK within the specified timeout period:
- Receiver’s Handling of Retransmission:
- If the receiver receives a retransmitted data packet, it performs error detection again.
- If the data packet is error-free, the receiver sends an ACK.
- If the data packet is still corrupted or lost, the receiver sends a NAK again.
- Looping Process:
- The above steps are repeated until all data packets are successfully transmitted and acknowledged.
- The sender keeps sending packets and waiting for corresponding acknowledgments.
- The receiver keeps receiving packets, checking for errors, and sending ACKs or NAKs as needed.
The Stop-and-Wait ARQ protocol ensures reliable data transmission by using acknowledgments and negative acknowledgments to handle errors. If the receiver detects an error in a received packet, it sends a NAK to request the sender to retransmit the packet. The sender, upon receiving a NAK or not receiving any acknowledgment within the timeout period, retransmits the packet.
The protocol’s stop-and-wait nature guarantees that the sender and receiver stay synchronized, as the sender waits for acknowledgments before proceeding with the next packet. While this ensures reliability, it also introduces inefficiencies and latency, particularly in scenarios with high network error rates or long propagation delays.
Implementing Stop-and-Wait ARQ Protocol in Java:
Server program:
import java.io.*;
import java.net.*;
public class Server {
public static void main(String[] args) {
try {
ServerSocket serverSocket = new ServerSocket(8000);
System.out.println("Server started. Waiting for a connection...");
Socket socket = serverSocket.accept();
System.out.println("Client connected.");
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
String data = in.readLine();
System.out.println("Received data: " + data);
// Process the received data
// Simulate random error occurrence
boolean isError = Math.random() < 0.2;
if (isError) {
System.out.println("Data packet corrupted. Sending NAK.");
out.println("NAK");
} else {
System.out.println("Data packet received successfully. Sending ACK.");
out.println("ACK");
}
socket.close();
serverSocket.close();
System.out.println("Connection closed.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
JavaClient program:
import java.io.*;
import java.net.*;
public class Client {
public static void main(String[] args) {
try {
Socket socket = new Socket("localhost", 8000);
System.out.println("Connected to server.");
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
String data = "Hello, Server!";
out.println(data);
System.out.println("Sent data: " + data);
String response = in.readLine();
if (response.equals("ACK")) {
System.out.println("Received ACK. Data packet delivered successfully.");
} else if (response.equals("NAK")) {
System.out.println("Received NAK. Data packet corrupted. Retransmitting...");
out.println(data); // Retransmit the data packet
response = in.readLine();
if (response.equals("ACK")) {
System.out.println("Received ACK. Retransmission successful.");
} else {
System.out.println("Retransmission failed. Data packet lost.");
}
}
socket.close();
System.out.println("Connection closed.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
JavaAdvantages:
- Reliability: The Stop-and-Wait ARQ protocol ensures reliable data transmission by implementing acknowledgments and retransmission mechanisms.
- Error Detection: The protocol incorporates error detection mechanisms, such as NAK responses, to identify and handle corrupted or lost data packets.
- Simplicity: Similar to the basic Stop and Wait protocol, Stop-and-Wait ARQ is relatively simple to implement and understand.
Drawbacks:
- Limited Throughput: The protocol’s stop-and-wait mechanism limits the overall throughput, as the sender has to wait for acknowledgments before sending the next data packet.
- Increased Latency: The inclusion of acknowledgments and potential retransmissions introduces additional latency into the communication process.
- Inefficient for High Error Rates: In scenarios with high error rates, the frequent retransmissions required by the protocol can lead to significant performance degradation.
The Stop-and-Wait ARQ protocol improves upon the basic Stop and Wait protocol by incorporating error detection and retransmission mechanisms. While it provides reliability and error handling, the protocol’s stop-and-wait nature can limit throughput and introduce latency, making it less efficient in certain scenarios. In the next section, we will explore another protocol, the Sliding Window protocol, which aims to address these limitations and optimize data transmission further.
The Sliding Window Protocol was introduced to overcome the limitations of the Stop-and-Wait ARQ protocol and improve the efficiency of data transmission. It allows multiple packets to be sent and acknowledged simultaneously, effectively increasing the throughput of the communication channel.
Now let’s delve into the detailed explanation of the Sliding Window Protocol:
Sliding Window Protocol:
The Sliding Window Protocol is a flow control protocol that enables efficient and reliable data transmission in computer networks. It divides the data into a series of fixed-size frames and assigns sequence numbers to each frame. The sender maintains a sliding window that represents the range of acceptable sequence numbers, while the receiver uses a similar sliding window to track the expected sequence numbers. This protocol ensures that the sender can transmit multiple frames without waiting for individual acknowledgments and that the receiver can correctly reorder and deliver the received frames.
The Sliding Window Protocol offers two main variations:
- Go-Back-N ARQ:
- Go-Back-N ARQ is a type of Sliding Window Protocol that provides a mechanism for handling errors and retransmissions.
- It allows the sender to transmit multiple frames without waiting for individual acknowledgments.
- If an error occurs or an acknowledgment is not received within a specified timeout, the sender retransmits all frames starting from the earliest unacknowledged frame.
- Selective Repeat ARQ:
- Selective Repeat ARQ is another variation of the Sliding Window Protocol that improves efficiency by allowing the receiver to acknowledge individual frames.
- The sender transmits multiple frames and maintains a window of unacknowledged frames.
- Upon receiving a frame, the receiver acknowledges the frame individually, indicating successful receipt.
- In case of an error, the receiver requests retransmission of only the corrupted frame, reducing unnecessary retransmissions.
The Sliding Window Protocol offers several advantages over the Stop-and-Wait ARQ protocol. It enhances throughput by allowing the sender to transmit multiple frames before waiting for acknowledgments, effectively utilizing the available bandwidth. The receiver can reorder out-of-sequence frames, further improving efficiency. However, the Sliding Window Protocol also introduces additional complexity and requires proper management of window sizes and acknowledgments.
Go-Back-N ARQ:
The Go-Back-N Automatic Repeat Request (ARQ) protocol is a type of Sliding Window Protocol that provides reliable data transmission between a sender and a receiver in a computer network. It allows the sender to transmit multiple frames without waiting for individual acknowledgments, improving efficiency. In case of errors or lost acknowledgments, the sender retransmits a window of frames starting from the earliest unacknowledged frame.
The Go-Back-N ARQ protocol involves the following steps:
- Sender Side:
- The sender divides the data into fixed-size frames and assigns sequence numbers to each frame.
- The sender maintains a sending window that represents the range of acceptable sequence numbers.
- The sender sends a window of frames to the receiver, starting from the base of the sending window.
- The sender starts a timer for the first frame in the window.
- Receiver Side:
- The receiver maintains a receiving window that represents the range of expected sequence numbers.
- The receiver receives frames from the sender.
- If a frame is received within the receiving window and is error-free, it is accepted and acknowledged.
- The receiver sends cumulative acknowledgments for the highest in-order frame received.
- Sender’s Handling of Acknowledgments and Timeouts:
- When the sender receives an acknowledgment, it updates the base of the sending window accordingly.
- The sender slides the sending window by advancing the base to the next unacknowledged frame.
- If the sender does not receive an acknowledgment within a specified timeout period, it assumes that an error or loss occurred.
- Upon timeout, the sender retransmits all frames in the current window, starting from the base.
The sender sends a window of frames, starts a timer, and waits for acknowledgments. The receiver receives the frames, checks for errors, and sends cumulative acknowledgments for the highest in-order frame received. If errors are detected or acknowledgments are not received within the timeout period, the sender retransmits the current window of frames.
Implementation of Go-Back-N ARQ Protocol:
Here’s an example of how the Go-Back-N ARQ protocol can be implemented using Java for the server and client programs. Please note that this is a simplified implementation and may require further customization based on specific requirements.
Sender (Client) Program:
import java.io.*;
import java.net.*;
public class Sender {
private static final int WINDOW_SIZE = 4;
private static final int TIMEOUT = 3000;
public static void main(String[] args) throws Exception {
// Create a socket and connect to the receiver
Socket socket = new Socket("localhost", 12345);
// Create input and output streams
DataInputStream in = new DataInputStream(socket.getInputStream());
DataOutputStream out = new DataOutputStream(socket.getOutputStream());
// Simulated data to be sent
byte[] data = "Hello, Receiver!".getBytes();
int base = 0;
int nextSeqNum = 0;
boolean[] ackReceived = new boolean[data.length];
while (base < data.length) {
// Send the window of frames
for (int i = base; i < Math.min(base + WINDOW_SIZE, data.length); i++) {
out.writeInt(i); // Send the sequence number
out.writeInt(data[i]); // Send the frame
ackReceived[i] = false; // Set acknowledgment status
}
// Start the timer
long startTime = System.currentTimeMillis();
// Receive acknowledgments
while (System.currentTimeMillis() - startTime < TIMEOUT) {
if (in.available() > 0) {
int ack = in.readInt(); // Receive acknowledgment
if (ack >= base && ack < base + WINDOW_SIZE) {
ackReceived[ack] = true; // Set acknowledgment status
if (ack == base) {
// Slide the window
while (base < data.length && ackReceived[base]) {
base++;
nextSeqNum++;
}
break;
}
}
}
}
}
// Close the socket
socket.close();
}
}
JavaReceiver (Server) Program:
import java.io.*;
import java.net.*;
public class Receiver {
private static final int WINDOW_SIZE = 4;
public static void main(String[] args) throws Exception {
// Create a server socket
ServerSocket serverSocket = new ServerSocket(12345);
// Accept a client connection
Socket socket = serverSocket.accept();
// Create input and output streams
DataInputStream in = new DataInputStream(socket.getInputStream());
DataOutputStream out = new DataOutputStream(socket.getOutputStream());
while (true) {
if (in.available() > 0) {
int seqNum = in.readInt(); // Receive the sequence number
int frame = in.readInt(); // Receive the frame
// Simulate frame error (e.g., uncomment the line below)
// if (seqNum == 2) continue;
// Simulate frame loss (e.g., uncomment the line below)
// if (seqNum == 3) continue;
if (seqNum >= 0 && seqNum < WINDOW_SIZE) {
// Process the frame
System.out.println("Received frame with sequence number: " + seqNum);
System.out.println("Frame data: " + frame);
// Send acknowledgment
out.writeInt(seqNum);
}
}
}
}
}
JavaPlease note that this code provides a simplified implementation of the Go-Back-N ARQ protocol and may require further enhancements and error handling for a production environment.
Advantages:
- Improved efficiency compared to the Stop-and-Wait protocol by allowing the sender to transmit multiple frames before waiting for acknowledgments.
- Utilizes the available bandwidth more effectively by overlapping data transmission and acknowledgment.
- Provides automatic retransmission of lost or corrupted frames, ensuring reliability.
Drawbacks:
- Increased complexity in managing the sending and receiving windows and handling acknowledgments.
- The retransmission of the entire window upon error or loss can lead to unnecessary retransmissions, affecting efficiency.
- Limited scalability in high-error or high-latency networks due to the potential for frequent retransmissions.
The Go-Back-N ARQ protocol improves data transmission efficiency by allowing the sender to transmit multiple frames without waiting for individual acknowledgments. However, it introduces complexity and may not be suitable for networks with high error rates or long propagation delays.
Selective Repeat ARQ Protocol:
The Selective Repeat Automatic Repeat Request (ARQ) protocol is another type of Sliding Window Protocol that provides reliable data transmission between a sender and a receiver in a computer network. It allows the sender to transmit multiple frames without waiting for acknowledgments and selectively retransmits only the frames that are lost or damaged. The receiver acknowledges each correctly received frame individually.
The Selective Repeat ARQ protocol involves the following steps:
- Sender Side:
- The sender divides the data into fixed-size frames and assigns sequence numbers to each frame.
- The sender maintains a sending window that represents the range of acceptable sequence numbers.
- The sender sends a window of frames to the receiver, starting from the base of the sending window.
- The sender starts a timer for the first frame in the window.
- Receiver Side:
- The receiver maintains a receiving window that represents the range of expected sequence numbers.
- The receiver receives frames from the sender.
- If a frame is received within the receiving window and is error-free, it is accepted and acknowledged individually.
- The receiver sends an acknowledgment for each correctly received frame.
- Sender’s Handling of Acknowledgments and Timeouts:
- When the sender receives an acknowledgment, it updates the base of the sending window and marks the corresponding frame as acknowledged.
- The sender slides the sending window by advancing the base to the next unacknowledged frame.
- If the sender does not receive an acknowledgment within a specified timeout period, it assumes that an error or loss occurred.
- Upon timeout, the sender retransmits only the unacknowledged frames within the sending window.
Implementation of Selective Repeat ARQ Protocol:
Here’s an example of how the Selective Repeat ARQ protocol can be implemented using Java for the server and client programs. Please note that this is a simplified implementation and may require further customization based on specific requirements.
Sender (Client) Program:
import java.io.*;
import java.net.*;
public class Sender {
private static final int WINDOW_SIZE = 4;
private static final int TIMEOUT = 3000;
public static void main(String[] args) throws Exception {
// Create a socket and connect to the receiver
Socket socket = new Socket("localhost", 12345);
// Create input and output streams
DataInputStream in = new DataInputStream(socket.getInputStream());
DataOutputStream out = new DataOutputStream(socket.getOutputStream());
// Simulated data to be sent
byte[] data = "Hello, Receiver!".getBytes();
int base = 0;
int nextSeqNum = 0;
boolean[] ackReceived = new boolean[data.length];
while (base < data.length) {
// Send the window of frames
for (int i = base; i < Math.min(base + WINDOW_SIZE, data.length); i++) {
out.writeInt(i); // Send the sequence number
out.writeInt(data[i]); // Send the frame
ackReceived[i] = false; // Set acknowledgment status
}
// Start the timer
long startTime = System.currentTimeMillis();
// Receive acknowledgments
while (System.currentTimeMillis() - startTime < TIMEOUT) {
if (in.available() > 0) {
int ack = in.readInt(); // Receive acknowledgment
if (ack >= base && ack < base + WINDOW_SIZE) {
ackReceived[ack] = true; // Set acknowledgment status
if (ack == base) {
// Slide the window
while (base < data.length && ackReceived[base]) {
base++;
nextSeqNum++;
}
break;
}
}
}
}
}
// Close the socket
socket.close();
}
}
JavaReceiver (Server) Program:
import java.io.*;
import java.net.*;
public class Receiver {
private static final int WINDOW_SIZE = 4;
public static void main(String[] args) throws Exception {
// Create a server socket
ServerSocket serverSocket = new ServerSocket(12345);
// Accept a client connection
Socket socket = serverSocket.accept();
// Create input and output streams
DataInputStream in = new DataInputStream(socket.getInputStream());
DataOutputStream out = new DataOutputStream(socket.getOutputStream());
while (true) {
if (in.available() > 0) {
int seqNum = in.readInt(); // Receive the sequence number
int frame = in.readInt(); // Receive the frame
// Simulate frame error (e.g., uncomment the line below)
// if (seqNum == 2) continue;
// Simulate frame loss (e.g., uncomment the line below)
// if (seqNum == 3) continue;
if (seqNum >= 0 && seqNum < WINDOW_SIZE) {
// Process the frame
System.out.println("Received frame with sequence number: " + seqNum);
System.out.println("Frame data: " + frame);
// Send acknowledgment
out.writeInt(seqNum);
}
}
}
}
}
JavaAdvantages:
- Improved efficiency compared to the Stop-and-Wait protocol by allowing the sender to transmit multiple frames before waiting for acknowledgments.
- Selective retransmission reduces unnecessary retransmissions, improving overall network efficiency.
- Provides greater flexibility in handling out-of-order or lost frames.
Drawbacks:
- Increased complexity in managing the sending and receiving windows and handling acknowledgments.
- Requires additional buffer space at the receiver to store out-of-order frames until they can be delivered to the upper layers.
- May suffer from increased latency if acknowledgments are lost or delayed, as the sender has to wait for individual acknowledgments.
The Selective Repeat ARQ protocol enhances the efficiency of data transmission by allowing the sender to transmit multiple frames without waiting for individual acknowledgments. However, it introduces complexity and requires additional buffer space, making it more suitable for reliable networks where out-of-order delivery and frame losses are common.
Comparison and Trade-offs:
When comparing the Stop-and-Wait ARQ, Go-Back-N ARQ, and Selective Repeat ARQ protocols, it’s essential to consider their advantages, drawbacks, and trade-offs. Here’s a comparison table highlighting key aspects of these protocols:
Aspect | Stop-and-Wait ARQ | Go-Back-N ARQ | Selective Repeat ARQ |
---|---|---|---|
Efficiency | Low | Moderate | High |
Throughput | Low | Moderate | High |
Acknowledgment Overhead | High | Moderate | Moderate to High |
Retransmission Overhead | High | High | Low to Moderate |
Buffer Requirement | Low | Low to Moderate | Moderate to High |
Error Recovery | Limited | All or None | Selective |
Network Utilization | Low | Moderate | High |
Sequence Number Size | 1 bit | N bits | N bits |
Complexity | Low | Moderate | High |
Trade-offs:
- Efficiency and Throughput:
- Stop-and-Wait ARQ has the lowest efficiency and throughput due to its one-frame-at-a-time transmission and strict acknowledgment waiting.
- Go-Back-N ARQ offers moderate efficiency and throughput by allowing multiple frames to be sent before receiving acknowledgments, but retransmissions can lead to lower efficiency.
- Selective Repeat ARQ provides higher efficiency and throughput by selectively retransmitting only lost or damaged frames while accepting correctly received frames.
- Acknowledgment and Retransmission Overhead:
- Stop-and-Wait ARQ incurs high acknowledgment overhead as each frame requires an individual acknowledgment.
- Go-Back-N ARQ has moderate acknowledgment overhead since cumulative acknowledgments are used.
- Selective Repeat ARQ also has moderate to high acknowledgment overhead as acknowledgments are sent individually for each frame.
- Buffer Requirement:
- Stop-and-Wait ARQ requires a minimal buffer at the receiver as only one frame is expected at a time.
- Go-Back-N ARQ needs a buffer to store out-of-order frames until missing frames arrive for reassembly.
- Selective Repeat ARQ demands a larger buffer to store out-of-order frames until they can be delivered to the upper layers.
- Error Recovery:
- Stop-and-Wait ARQ has limited error recovery capabilities since it requires retransmission of the entire frame in case of an error.
- Go-Back-N ARQ provides all-or-none error recovery, where a single error leads to retransmission of multiple frames.
- Selective Repeat ARQ offers selective error recovery by retransmitting only the lost or damaged frames.
- Network Utilization:
- Stop-and-Wait ARQ underutilizes the network due to frequent waiting for acknowledgments.
- Go-Back-N ARQ achieves moderate network utilization by allowing the sender to send multiple frames before waiting for acknowledgments.
- Selective Repeat ARQ maximizes network utilization by selectively retransmitting only necessary frames.
- Complexity:
- Stop-and-Wait ARQ has the lowest complexity as it involves straightforward frame transmission and acknowledgment handling.
- Go-Back-N ARQ introduces moderate complexity due to the need for sequence numbers, cumulative acknowledgments, and buffer management.
- Selective Repeat ARQ has the highest complexity with the management of sending and receiving windows, individual acknowledgments, and out-of-order frame handling.
By considering these factors, network designers can make informed decisions about the appropriate ARQ protocol to use based on the specific requirements of their communication system.
ARQ Strategies in Different Scenarios:
In different network scenarios, the choice of ARQ (Automatic Repeat Request) strategies can vary based on factors such as network conditions, reliability requirements, and performance considerations. Let’s explore the use of different ARQ strategies in various scenarios:
- Low Error Rate and Reliable Network:
In a scenario where the network has a low error rate and is highly reliable, a simple and efficient ARQ strategy like Stop-and-Wait ARQ may be sufficient. Its straightforward implementation and low complexity make it suitable for such scenarios, without the need for additional overhead and complexity introduced by other ARQ strategies. - Noisy or Unreliable Network:
In situations where the network is prone to errors or is unreliable, more robust ARQ strategies like Go-Back-N ARQ or Selective Repeat ARQ are commonly used. These strategies provide error recovery mechanisms by retransmitting lost or damaged frames. Go-Back-N ARQ is suitable when errors are bursty and consecutive, as it allows for the retransmission of a window of frames upon detection of an error. Selective Repeat ARQ, on the other hand, offers more selective retransmissions, minimizing retransmission overhead and enhancing network efficiency. - Delay-Sensitive Applications:
For applications that are highly sensitive to delays, such as real-time multimedia streaming or voice over IP (VoIP) communication, the choice of ARQ strategy becomes critical. Stop-and-Wait ARQ, despite its simplicity, introduces significant delays due to the waiting time for acknowledgments. In such scenarios, Go-Back-N ARQ or Selective Repeat ARQ can be used to achieve higher throughput and reduced latency by allowing the sender to transmit multiple frames before waiting for acknowledgments. - High Bandwidth Networks:
In scenarios where the network has high bandwidth capabilities, the impact of retransmissions on overall performance and efficiency becomes more significant. Selective Repeat ARQ is advantageous in such situations as it minimizes retransmission overhead by selectively retransmitting only the necessary frames. This approach helps maximize network utilization and optimize bandwidth usage. - Wireless Networks:
Wireless networks often face challenges such as fading, interference, and varying channel conditions. In these scenarios, hybrid ARQ strategies are commonly employed. Hybrid ARQ combines the benefits of ARQ protocols with forward error correction (FEC) techniques. It allows for error detection and correction at the receiver while using ARQ for retransmissions when necessary. This combination improves reliability and performance in wireless networks.
It’s important to note that the choice of ARQ strategy depends on a thorough understanding of the specific network environment, reliability requirements, latency constraints, and available bandwidth. Network designers and engineers must evaluate these factors to select the most appropriate ARQ strategy for a given scenario, ensuring optimal performance and reliability.
Conclusion:
In the realm of socket programming, understanding protocols is crucial for building reliable and efficient network communication systems. In this blog, we explored two main protocols used in socket programming: the Stop-and-Wait Protocol and the Sliding Window Protocol.
Each protocol has its advantages and drawbacks, and the choice depends on specific network requirements. Stop-and-Wait ARQ is suitable for low-error-rate scenarios, while Go-Back-N ARQ and Selective Repeat ARQ are preferred in noisy or unreliable networks. Moreover, the choice of protocol is influenced by factors such as delay sensitivity, bandwidth availability, and wireless network conditions.
It is important for network designers and engineers to carefully consider these protocols and their trade-offs to optimize the performance, reliability, and efficiency of their socket programming applications. By selecting the appropriate protocol and implementing it effectively, reliable data transmission can be achieved, meeting the demands of diverse network scenarios.
Socket programming and protocols continue to play a crucial role in modern networking systems, enabling efficient communication and data transfer across various domains. By understanding and utilizing these protocols effectively, developers can build robust and scalable applications that deliver seamless and reliable network communication experiences.