Follow Us:
How to Use the wp_insert_post Function in WordPress to Insert Custom Posts?
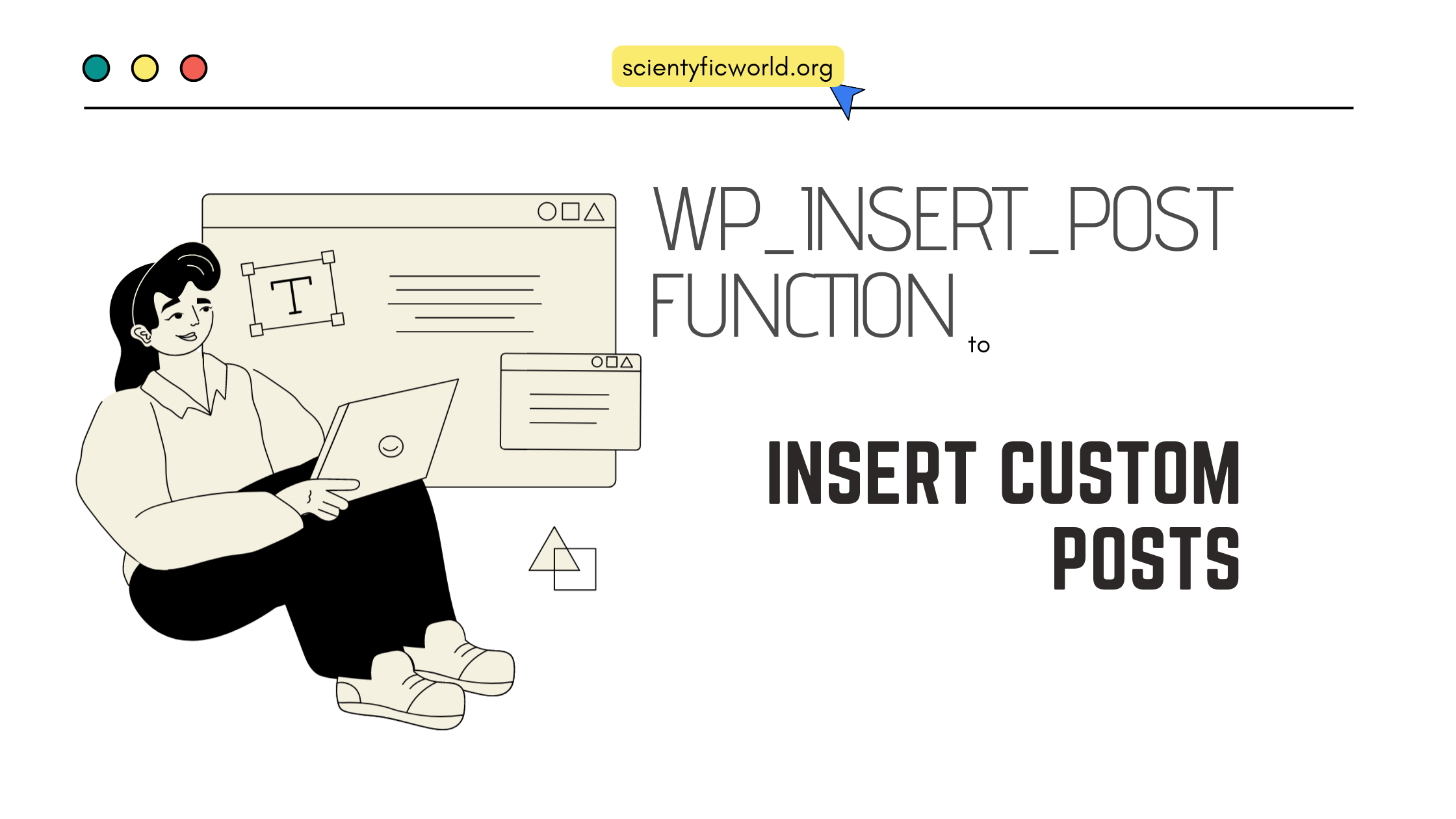
In this guide, we will explore the power of the wp_insert_post
function, its parameters, and how to leverage it effectively. By the end of this article, you’ll have a clear understanding of how to use this function to streamline your post-creation process and enhance your WordPress site’s functionality.
We will start by discussing why using this function is beneficial and explore its various parameters, providing insights into how each parameter influences the post-creation process. Additionally, we will provide a step-by-step guide on how to use the wp_insert_post
function, covering topics such as inserting posts, checking for errors, interacting with the WordPress database, and inserting posts with a custom taxonomy and post metadata. By the end, you will have the knowledge and skills necessary to effectively utilize the wp_insert_post
function and optimize your WordPress website’s post management capabilities.
Workflow of the wp_insert_post Function:
The wp_insert_post
function in WordPress follows a structured workflow to insert new posts into the WordPress database. Understanding the inner functionality and workflow of this function can provide insights into how it works. Here is a breakdown of the workflow:
- Data Validation and Sanitization:
- When called, the wp_insert_post function first validates and sanitizes the data provided as parameters.
- It ensures that the required data, such as the post title and content, is present and properly formatted.
- Data sanitization helps prevent security vulnerabilities and ensures the integrity of the post data.
- Preparing the Post Object:
- Once the data is validated, the function prepares a post object that contains all the necessary information for the new post.
- The post object includes properties such as post title, content, author, status, post type, and more.
- Additional optional parameters, such as post excerpt, post parent, or custom meta data, can also be included in the post object.
- Applying Filters:
- Before the post is inserted into the database, the wp_insert_post function applies a series of filters to allow customization and modification of the post data.
- These filters enable developers to manipulate the post object or modify specific properties based on their requirements.
- Filter hooks such as ‘wp_insert_post_data’ and ‘wp_insert_post_empty_content’ provide opportunities for customizing the post data.
- Inserting the Post:
- With the validated and filtered post object, the wp_insert_post function proceeds to insert the post into the WordPress database.
- It utilizes the underlying database functions to create a new entry in the ‘wp_posts’ table.
- The function assigns a unique post ID to the newly created post, which serves as its identifier.
- Performing Actions:
- After the post is successfully inserted, the wp_insert_post function triggers a series of actions and hooks, allowing other plugins or themes to perform additional tasks.
- Actions such as ‘save_post’, ‘publish_post’, or ‘wp_insert_post’ can be hooked into for executing custom code related to the inserted post.
- These actions provide opportunities for extending the functionality and integrating with other parts of the WordPress ecosystem.
By following this workflow, the wp_insert_post function ensures the proper creation and insertion of new posts into the WordPress database. Understanding the inner workings of this function empowers developers to utilize it effectively and extend its functionality through the use of filter hooks and actions.
Why Use the wp_insert_post Function?
The wp_insert_post
function is a powerful tool that brings numerous benefits to WordPress site owners and developers. Here are some reasons behind using this function:
- Seamless Post Insertion:
The primary purpose of thewp_insert_post
function is to simplify the process of inserting posts into your WordPress site. Instead of manually creating posts through the WordPress admin interface, the function enables you to programmatically insert posts directly from your code. This not only saves time but also allows for greater control and flexibility over the content creation process. - Automation and Workflow Efficiency:
With thewp_insert_post
function, you can automate the creation of posts on your WordPress site. This is particularly useful if you have a large number of posts to import or if you need to regularly update your site with new content. By utilizing the function, you can streamline your workflow, reduce manual effort, ensure consistency in post formatting and structure, and schedule the article publishing process. - Customization and Dynamic Content:
Thewp_insert_post
function empowers you to generate customized posts tailored to your specific requirements. You can define various post parameters such as the post title, content, author, categories, tags, and more. This level of customization allows you to create dynamic and engaging content that resonates with your target audience. - Integration with Plugins and Themes:
Thewp_insert_post
function seamlessly integrates with WordPress plugins and themes, expanding its capabilities even further. Many plugins and themes rely on the wp_insert_post function to provide advanced features, such as importing content, generating custom post types, or performing complex post manipulations. By utilizing the function, you can tap into the extended functionality offered by these plugins and themes. - Scalability and Bulk Operations:
For websites with a substantial amount of content, thewp_insert_post
function becomes an invaluable tool for handling bulk operations efficiently. Whether you’re migrating content from another platform, importing data from a CSV file, or synchronizing content across multiple sites, the function allows you to insert a large number of posts quickly and effectively.
Now that we understand the benefits of using the wp_insert_post function, let’s delve into its parameters, which define the properties of the posts you insert. In the next section, we will explore the various parameters supported by the wp_insert_post
function and their significance.
Parameters for wp_insert_post
The wp_insert_post
function accepts an array, $postarr
, which contains various elements defining the properties of the post to be inserted or updated. Let’s explore each parameter and its role within the function.
// Sample code for using the wp_insert_post function
$postarr = array(
'ID' => 0,
'post_author' => get_current_user_id(),
'post_date' => current_time('mysql'),
'post_date_gmt' => get_gmt_from_date( current_time('Y-m-d H:i:s') ),
'post_content' => '',
'post_content_filtered' => '',
'post_title' => '',
'post_excerpt' => '',
'post_status' => 'draft',
'post_type' => 'post',
'comment_status' => get_option( 'default_comment_status' ),
'ping_status' => get_option( 'default_ping_status' ),
'post_password' => '',
'post_name' => sanitize_title('', '', 'save'),
'to_ping' => '',
'pinged' => '',
'post_parent' => 0,
'menu_order' => 0,
'post_mime_type' => '',
'guid' => '',
'import_id' => 0,
'post_category' => array( get_option( 'default_category' ) ),
'tags_input' => array(),
'tax_input' => array(),
'meta_input' => array(),
'page_template' => '',
'wp_error' => false,
'fire_after_hooks' => true
);
// Insert the post using the wp_insert_post function
$new_post_id = wp_insert_post( $postarr );
PHPHow to Use the wp_insert_post Function in WordPress?
Now that we have a thorough understanding of the parameters, let’s dive into the step-by-step process of using the wp_insert_post
function to insert custom posts in WordPress.
Here is a sample code to use the function:
<form method="POST" action="">
<div>
<label for="post_title">Post Title:</label>
<input type="text" id="post_title" name="post_title" required>
</div>
<div>
<label for="post_content">Post Content:</label>
<textarea id="post_content" name="post_content" required></textarea>
</div>
<button type="submit" name="submit">Create Post</button>
</form>
HTML<?php
// Example of using the wp_insert_post function with user input
if ( isset( $_POST['submit'] ) ) {
$post_title = sanitize_text_field( $_POST['post_title'] );
$post_content = wp_kses_post( $_POST['post_content'] );
$post_author = get_current_user_id(); // Use the current logged-in user as the author
$post_status = 'publish';
$post_type = 'post';
$post_data = array(
'post_title' => $post_title,
'post_content' => $post_content,
'post_status' => $post_status,
'post_author' => $post_author,
'post_type' => $post_type
);
// Insert the post into the database
$post_id = wp_insert_post( $post_data );
if ( $post_id ) {
echo 'Custom post inserted successfully with ID: ' . $post_id;
} else {
echo 'Error inserting custom post.';
}
}
?>
Function.phpThe isset()
function checks if the “submit” button in the form has been clicked or not. If it has been clicked, the code within the if
statement will be executed. This helps ensure that the post data is only processed when the form is submitted.
Inside the if
loop, the code retrieves the values of post_title
and post_content
from the form submission using $_POST
. It sanitizes the input using appropriate functions (sanitize_text_field
and wp_kses_post
) to ensure data security.
Then, it sets the author, status, and post type for the new post by assigning values to variables.
Finally, the wp_insert_post
function is called to insert the post into the WordPress database using the prepared data. If the post is inserted successfully, it will display a success message with the post ID. Otherwise, an error message will be displayed.
Sample video:
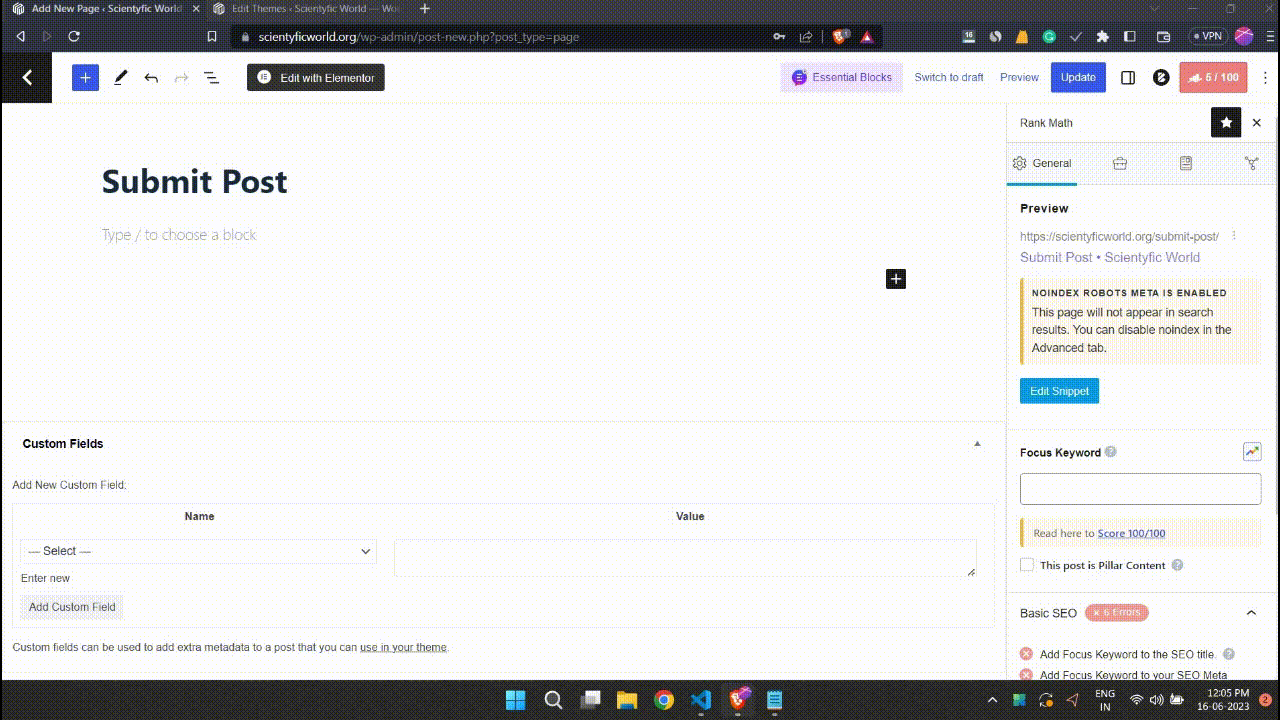
Now, let’s see some more advanced steps to insert a post using the wp_insert_post
function.
1. How to Insert a Post and Check for Errors
To insert a post using the wp_insert_post
function, follow these steps:
- Set up the post data
- Define an array,
$postarr
, containing the necessary post parameters such aspost_title
,post_content
,post_status
, and more. - Assign values to the desired parameters based on your requirements.
- Define an array,
- Insert the post
- Call the wp_insert_post function with the $postarr array as the argument.
- The function will return the post ID of the inserted post.
- Check for errors
- After inserting the post, it’s crucial to check for any errors that may have occurred during the insertion process.
- Use conditional statements or error-handling mechanisms to validate the success of the post-insertion.
2. How to Insert a New Post Into a WordPress Database
If you need to insert a new post into the WordPress database, follow these steps:
- Prepare the post data
- Create an array, $postarr, and populate it with the necessary post details such as
post_title
,post_content
,post_status
, and more. - Set the appropriate values for each parameter based on your requirements.
- Create an array, $postarr, and populate it with the necessary post details such as
- Insert the post into the database
- Use the
wp_insert_post
function, passing the$postarr
array as the argument. - The function will insert the post into the WordPress database and return the post ID.
- Use the
- Verify the post insertion
- To ensure successful insertion, you can check if the returned post ID is greater than 0, indicating a valid insertion.
- Additionally, you can retrieve the inserted post using functions like
get_post
orget_permalink
and verify its existence.
3. How to Insert a Post With Custom Taxonomy and Post Meta Data
If you want to insert a post with custom taxonomy terms and post metadata, follow these steps:
- Set up the post data
- Create an array, $postarr, and include the required post parameters such as post_title, post_content, post_status, and more.
- Set the values for each parameter according to your needs.
- Add custom taxonomy terms
- Include the
tax_input
parameter in the$postarr
array. - Provide an array of taxonomy names as keys and the corresponding term names, slugs, or IDs as values.
- Ensure that you correctly match hierarchical taxonomies with term IDs separated by commas.
- Include the
- Add post metadata
- Use the meta_input parameter in the $postarr array.
- Specify an array of meta keys as keys and the corresponding meta values as values.
- This allows you to associate additional custom data with the inserted post.
wp_insert_post Hooks:
The wp_insert_post
function in WordPress provides several hooks that allow developers to extend and customize its behavior. These hooks enable you to modify post data, perform additional actions, or integrate with other plugins and themes during the post insertion process. Understanding these hooks can empower you to tailor the behavior of wp_insert_post
to suit your specific needs. Here are some important hooks associated with wp_insert_post
:
wp_insert_post_data
:- This filter hook allows you to modify the post data before it is inserted into the database.
- You can access and modify the
$data
parameter, which contains the post object data that will be inserted. - Examples of modifications include changing the post title, modifying the content, or updating post meta data.
wp_insert_post_empty_content
:- This filter hook is triggered when wp_insert_post encounters an empty post content.
- You can use this hook to customize the behavior when an empty post content is detected.
- For instance, you could prevent the post from being inserted, display a custom error message, or take alternative actions.
save_post
:- This action hook is triggered after a post is inserted or updated in the database.
- It allows you to perform additional tasks or modify post-related data after the post has been saved.
- You can use this hook to trigger custom functionality such as sending notifications, updating related data, or performing post-processing tasks.
publish_post
:- This action hook is fired when a post transitions to the “publish” status.
- It provides an opportunity to perform specific actions when a post becomes published.
- You can use this hook to trigger actions like clearing caches, notifying subscribers, or updating external services with the published content.
wp_insert_post_parent
:- This filter hook allows you to modify the parent ID of a post before it is inserted into the database.
- You can use this hook to dynamically assign or change the parent post for the newly inserted post.
- It can be useful for creating hierarchical relationships between posts or organizing content within your WordPress site.
These hooks, along with others associated with wp_insert_post
, offer flexibility and customization options to enhance the behavior and functionality of the wp_insert_post
function. By utilizing these hooks, you can extend the capabilities of wp_insert_post
, integrate with third-party plugins, and perform additional tasks to meet your specific requirements.
When using these hooks, it’s essential to refer to the WordPress Developer Documentation for detailed information on hook parameters, best practices, and any additional considerations.
Conclusion:
In conclusion, this article has provided a comprehensive guide on how to use the wp_insert_post
function in WordPress. We have explored the importance of this function and discussed its parameters, which allow for flexible customization of the inserted posts. By understanding how to utilize this function, WordPress users can efficiently manage and insert custom posts on their websites.
Throughout the article, we have covered the step-by-step process of inserting posts, checking for errors, inserting posts into the WordPress database, and including custom taxonomy and post metadata. By following these guidelines and leveraging the power of the wp_insert_post
function, users can streamline their post-management tasks and enhance their website’s functionality.