The printf() function is a standard library function in the C programming language that is used to display text on the screen. It is one of the most widely used functions in C programming and is used to print messages, variables, and other data types. The printf() function is a powerful tool that allows developers to control the output format of their programs. However, many developers may not be aware of the intricacies of the printf() function and may not know how to write their own printf() function.
The purpose of writing your own printf() function is to gain a deeper understanding of how the printf() function works and to have more control over the output of your programs. Additionally, writing your own printf() function can also be a great learning experience for those who are new to C programming.
In this article, we will go through the process of writing your own printf() function in C. We will start by understanding the format specifiers that are used in the printf() function, then we will move on to implementing the function. We will also cover testing and debugging techniques to ensure that the function works correctly. By the end of this article, you will have a solid understanding of how the printf() function works and will be able to write your own printf() function with confidence.
Now, here is a prior note:
It is not impossible to create a function like printf() without the help of the printf() function, but it would be a non-trivial task. printf() is a complex function that can handle a wide variety of data types and format specifiers, and it is implemented in C's standard library. The printf() implementation in C is not only used for printing but also it's used for formatting the string, so it would be a difficult task to replicate the functionality and performance of printf() in C, It would be much simpler to call the existing printf() function and use its functionality, rather than re-implementing it from scratch. In conclusion, it is possible to create a function like printf() without the help of the printf() function, but it would be a difficult and time-consuming task, and the existing printf() function provides a reliable and well-tested implementation. So, here we're making a function to only use the printing functionality of the printf() function.
Understanding the Format Specifiers:
The printf() function uses format specifiers to control the output format of the text that is being printed. A format specifier is a special character that is used to indicate the type of data that is being printed. For example, the %d format specifier is used to print integers, while the %s format specifier is used to print strings.
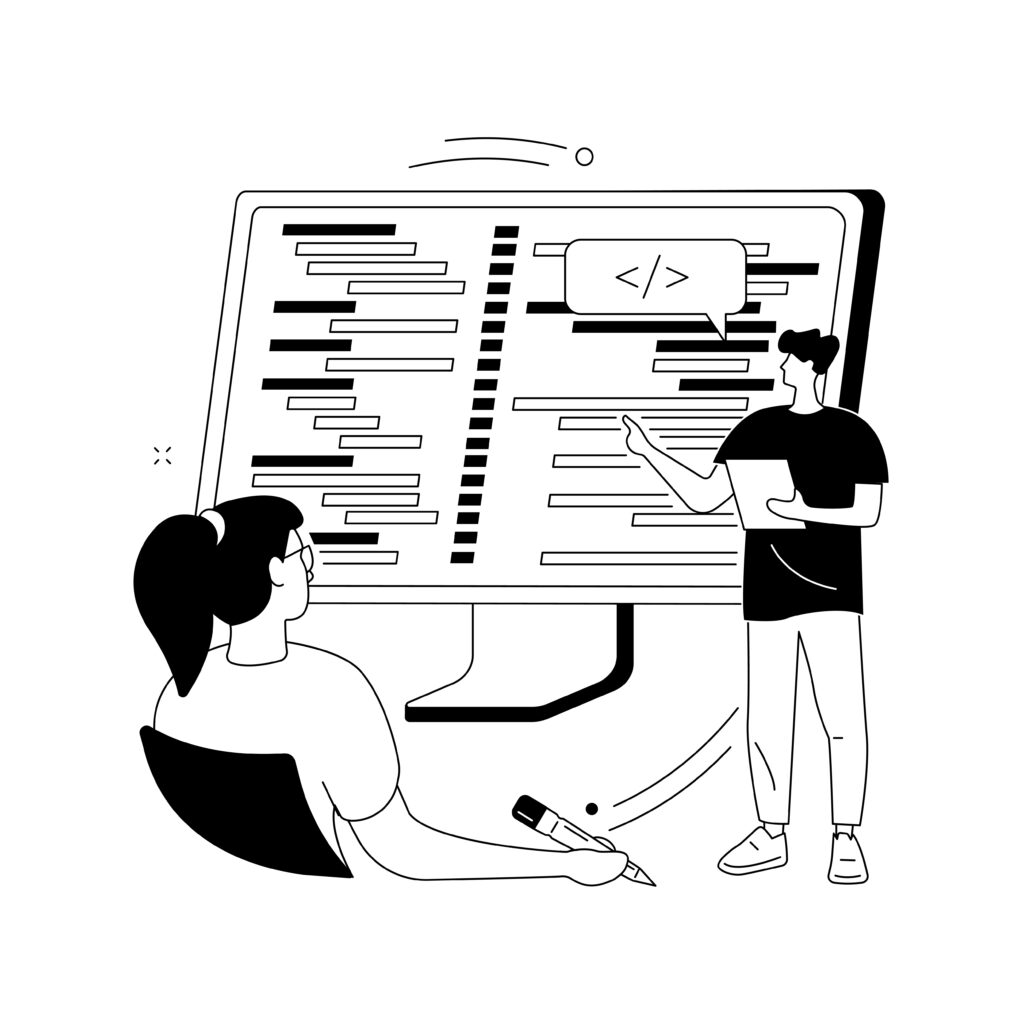
There are several types of format specifiers that can be used in the printf() function, including:
- %d or %i: Used to print integers
- %f: Used to print floating point numbers
- %c: Used to print characters
- %s: Used to print strings
- %x or %X: Used to print hexadecimal numbers
- %p: Used to print pointers
- %%: Used to print the % character
It is important to note that the format specifiers must be preceded by the % symbol, and must be followed by the appropriate argument. For example, if you want to print an integer, you would use the %d format specifier and provide the integer as an argument.
Understanding the format string is also crucial when it comes to writing your own printf() function. The format string is the first argument passed to the printf() function, and it contains the text that is to be printed along with the format specifiers.
For example, consider the following printf() function call:
printf("The value of x is %d", x);
In this example, “The value of x is %d” is the format string and x is the argument that corresponds to the %d format specifier.
In the next section, we will move on to implementing the printf() function.
Implementing the printf() function:
In this section, we will go over the process of implementing the printf() function. We will start by creating the function prototype and then move on to writing the function body. The function body will handle the different types of format specifiers and handle variable arguments using va_list, va_start and va_end macros.
Overview:
The implementation of the printf() function starts by creating the function prototype, that declares the function name, return type, and the number and type of its parameters. The next step is to write the function body, which handles the different types of format specifiers and variable arguments.
Creating the function prototype:
The first step in implementing the printf() function is to create the function prototype. The function prototype is a statement that declares the function’s name, return type, and the number and type of its parameters. The prototype for the printf() function is as follows:
int my_printf(const char *format, ...);
CIn this prototype, the function name is “my_printf”, the return type is int, and the function takes two parameters, the first one is a pointer to a constant character and the second one is variable arguments (indicated by the “…”).
Writing the function body:
Once the prototype is created, we can move on to writing the function body. The function body is where the actual code for the function is written. The main task of the function body is to handle the different types of format specifiers and to output the appropriate text.
Handling the different types of format specifiers:
The printf() function must be able to handle all the different types of format specifiers that can be used. To do this, we can use a switch statement to check for each format specifier and handle it accordingly. For example, if the format specifier is %d, we can use the printf() function to print an integer. If the format specifier is %s, we can use the printf() function to print a string. To do this, we must check the format string for the format specifier and take the appropriate action.
Handling variable arguments:
The printf() function takes variable arguments, which means that the number of arguments passed to the function can vary. To handle variable arguments, we can use the va_list, va_start, and va_end macros. These macros are used to create a list of variable arguments, start the list, and end the list, respectively. This allows us to handle any number of arguments passed to the function. And these macros comes from the library header <stdarg.h>
.
What is <stdarg.h>
?
The <stdarg.h>
header file is a C standard library header that is used for handling variable arguments in functions. It defines a set of macros and type definitions that allow a function to accept a variable number of arguments. These macros and type definitions are used to create a list of variable arguments, start the list, and end the list.
The <stdarg.h>
header file contains the following macros:
- va_list: This is a type definition that represents a list of variable arguments.
- va_start: This macro initializes a va_list object, making it ready to access the variable arguments.
- va_arg: This macro retrieves the next argument from the va_list.
- va_end: This macro is used to clean up the va_list object after the variable arguments have been processed.
These macros are used together to handle variable arguments in a function. The va_start macro is used to initialize a va_list object and start the list of variable arguments, the va_arg macro is used to retrieve the next argument from the list, and the va_end macro is used to clean up the va_list object after the variable arguments have been processed.
In the case of writing the printf() function, <stdarg.h>
is included in the header file to make use of the va_list, va_start, and va_end macros in handling variable arguments in the function.
As a developer, it’s important to keep in mind that the implementation of the printf() function is not a straightforward task. It requires a good understanding of C programming and the intricacies of the printf() function. Additionally, it’s essential to thoroughly test and debug the code to ensure that it works correctly.
For example, the following code demonstrates how to handle variable arguments:
va_list args;
va_start(args, format);
int x = va_arg(args, int);
va_end(args);
This code creates a va_list called args, starts the list with the “format” parameter, takes the next argument as an integer assigns it to the variable x, and finally ends the list.
Now, here is the actual code to create your custom print function.
#include <stdio.h>
#include <stdarg.h>
int myprintf(const char *format, ...)
{
va_list args;
va_start(args, format);
int count = 0;
while (*format != '\0')
{
if (*format != '%')
{
putchar(*format);
count++;
}
else
{
// Handle format specifiers
switch (*++format)
{
case 'd':
count += fprintf(stdout, "%d", va_arg(args, int));
break;
case 'c':
count += fprintf(stdout, "%c", va_arg(args, char));
break;
case 's':
count += fprintf(stdout, "%s", va_arg(args, char *));
break;
case 'f':
count += fprintf(stdout, "%f", va_arg(args, double));
break;
default:
// Handle unknown format specifiers
putchar('%');
putchar(*format);
count += 2;
break;
}
}
format++;
}
va_end(args);
return count;
}
CThis is just one example of how you can write your own printf() function in C. It is important to note that this function is not as robust as the standard printf() function and may not handle all edge cases. However, it is a good starting point for understanding how the printf() function works and how to write your own version of it.
It's essential to note that this function is not a replacement of the standard library function, but it's a way to understand the implementation of the printf() function and how it works.
Testing and Debugging
Once the function is written, it is important to test it with different inputs to ensure that it works correctly. Testing can be done by calling the function with different format specifiers and arguments.
Testing the function with different inputs
Testing is an important step when writing your own printf() function, as it ensures that the function works correctly. It is important to test the function with different inputs, including different format specifiers and arguments. For example, you can test the function by passing different integers, floating-point numbers, characters, strings, hexadecimal numbers, and pointers as arguments. Additionally, you can also test the function with different combinations of format specifiers and arguments to ensure that it handles them correctly.
Here is an example of how you can test the function:
int main(void)
{
int age = 25;
myprintf("My name is %s and I am %d years old.\n", "Snehasish", age);
double pi = 3.14;
myprintf("The value of pi is %.2f.\n", pi);
// Print a date and time.
myprintf("%s %s\n", "Today is", __DATE__);
myprintf("%s %s\n", "The time is", __TIME__);
// Print a list of numbers.
int numbers[] = {1, 2, 3, 4, 5};
for (int i = 0; i < sizeof(numbers) / sizeof(numbers[0]); i++)
{
myprintf("%d\n", numbers[i]);
}
// Print a table of data.
myprintf("| Name | Age | Occupation |\n");
myprintf("| Snehasish | 25 | AI Engineer |\n");
myprintf("| Alice | 30 | Software Engineer |\n");
}
CIn this example, the function is being tested with four different types of arguments, an integer, a floating-point number, a character, and a string.
Output:
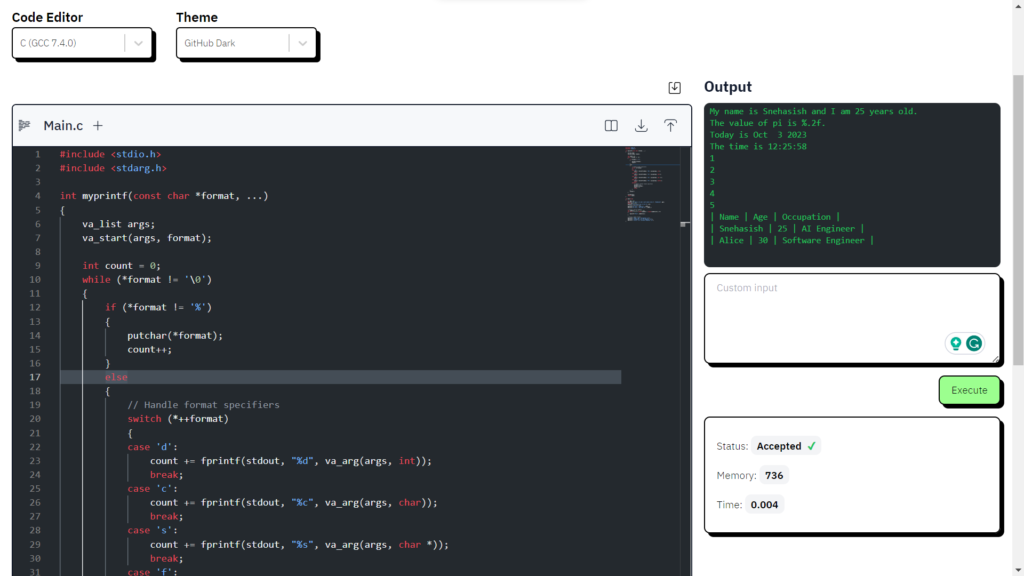
It is also important to test the function with edge cases. Edge cases are scenarios where the function might not work correctly. For example, if the function is not able to handle negative numbers, it would be important to test the function with negative numbers as well to ensure that it works correctly.
Debugging tips and techniques
Debugging is an important step when writing your own printf() function, as it helps to identify and fix errors in the code. Some common debugging techniques include:
- Using a debugger: A debugger is a tool that allows you to step through the code, line by line, and check the values of variables and memory locations. This can be helpful in identifying where the error occurs.
- Adding print statements: Adding print statements to the code can be helpful in identifying where the error occurs. For example, you can print the value of the format string and the arguments before the switch statement in the function body to check if they are being passed correctly.
- Using a linter: A linter is a tool that checks the code for errors and can be helpful in identifying syntax errors.
- Using assertions: Assertions are a way to test for a condition in the code, and if the condition is false, the program will stop and print an error message. This can be helpful in identifying when the function is not working as expected.
It’s also essential to keep in mind that debugging is an iterative process, and it may take some time to identify and fix the errors in the code. But with the right tools and techniques, it can be made more manageable.
It’s also important to test the function with different inputs and debug the code to ensure that it works correctly. With the right tools and techniques, you can write your own printf() function with confidence.
In conclusion, testing and debugging are crucial steps when writing your own printf() function. It is important to test the function with different inputs and debug the code to ensure that it works correctly. With the right tools and techniques, you can write your own printf() function with confidence.
Conclusion:
In this article, we have gone through the process of writing your own printf() function in C. We have covered the following topics:
- Understanding the format specifiers used in the printf() function
- Implementing the printf() function, including creating the function prototype, writing the function body, handling the different types of format specifiers, and handling variable arguments
- Testing and debugging techniques to ensure that the function works correctly
To write your own printf() function, you should have a clear understanding of the format specifiers and the format string, and how to handle variable arguments using va_list, va_start, and va_end macros.
It is important to test the function with different inputs and debug the code to ensure that it works correctly. With the right tools and techniques, you can write your own printf() function with confidence.
Additional resources for further learning include:
- The C Programming Language by Brian W. Kernighan and Dennis M. Ritchie
- The GNU C Library Reference Manual by Sandra Loosemore, Rachel Greenham, and Richard M. Stallman
These resources provide a more in-depth understanding of the C programming language and the standard library functions.
In conclusion, writing your own printf() function is a great way to gain a deeper understanding of the printf() function and the C programming language. It is a challenging task but with the right resources and techniques, it can be done with confidence.