Follow Us:
React Router DOM: A Developer’s Guide
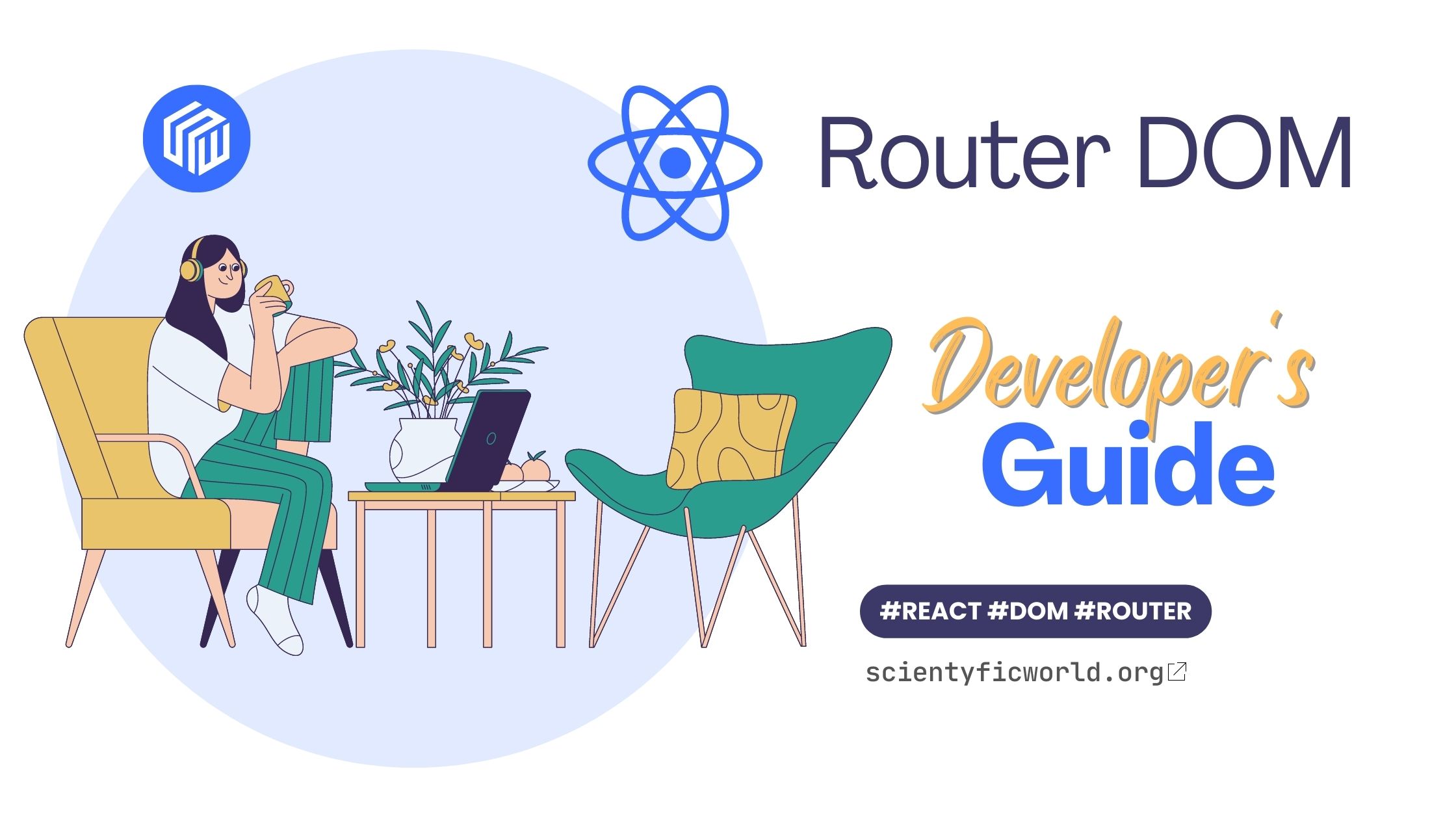
When building single page applications (SPA), it is common for there to be multiple routes that the user can take. React Router DOM is a routing package that allows developers to declaratively map routes to components. In this guide, we will take a look at how to use React Router DOM to create a basic routing setup.
But before starting, if you’re not familiar with the word DOM, then I prefer you to learn that first.
DOM:
The Document Object Model (DOM) is a programming interface for HTML, XML and SVG documents. It provides a structured representation of the document as a tree. The DOM defines methods that allow access to the tree, so that they can be changed.
The Document Object Model is a way of representing a document in a structure that can be read by computers. It is used by web browsers to access and manipulate web pages. The DOM is an essential part of how web pages are created and manipulated.
DOM tree structure:
The DOM is a tree-like structure that represents the structure of an HTML document. The DOM provides a way for programs to access and manipulate HTML elements, and is the foundation of most web development.
Each HTML element in a document is represented as a node in the DOM tree. Each node has a type, such as an element node, text node, or comment node. It also has properties such as its tag name, attributes, and child nodes.
For example, consider the following HTML code:
<html>
<head>
<title>Sample Document</title>
</head>
<body>
<h1>Hello World</h1>
<p>This is a sample document.</p>
</body>
</html>
HTMLThe corresponding DOM tree for this code would look like this:
html
/ \
head body
/ / \
title h1 p
|
This is a sample document.
MarkdownEach node in the DOM tree has a unique path from the root node (html
in this case) that can be used to access it using JavaScript. For example, to access the text content of the p
element, the following code can be used:
var pElement = document.body.getElementsByTagName("p")[0];
var pText = pElement.textContent;
JavaScriptThe DOM provides a rich set of methods and properties that allow developers to manipulate the HTML document dynamically. For example, the following code adds a new li
element to an existing ul
element:
var ulElement = document.body.getElementsByTagName("ul")[0];
var newLi = document.createElement("li");
newLi.textContent = "Item 4";
ulElement.appendChild(newLi);
JavaScriptWhat is React Router DOM?
React Router DOM is a routing package that enables developers to declaratively map routes to components. When React Router DOM is used in a React application, any route changes that are made will cause the React component hierarchy to be re-rendered. This allows for a seamless user experience as the user navigates through the application.
The router object has the following properties:
- location: A Location object that represents the current location.
- match: A Match object that represents the route that matched the location.
- history: A History object that can be used to navigate to different routes.
- React Router DOM also provides the following components:
- Route: A component that renders a component for a given route.
- Router: A component that manages the router state and renders the router.
- Link: A component that renders a link to a given route.
- Redirect: A component that redirects to a different route.
- Switch: A component that renders a component for the first matching route.
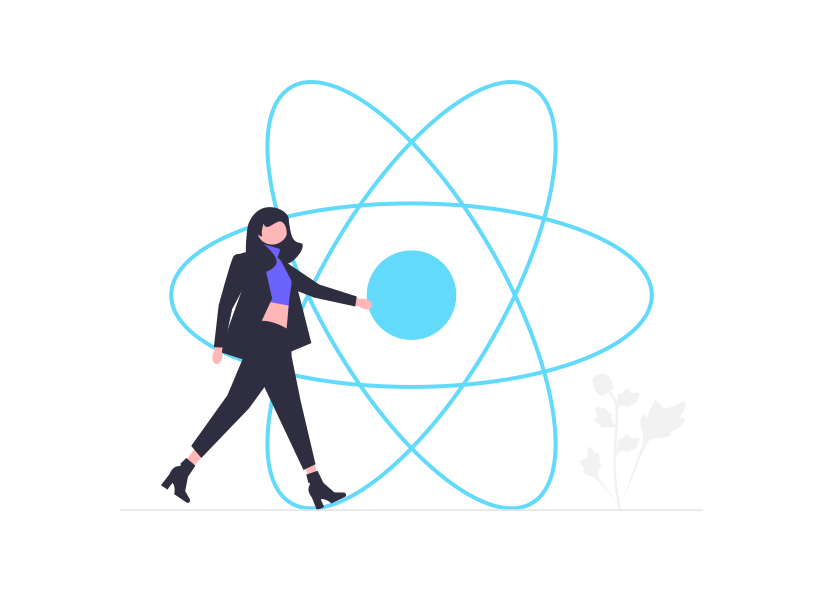
Now, React Router DOM can be used in both React and React Native applications. Let’s focus on using React Router DOM in a React application.
react-router-dom install:
Before we can start using React Router DOM, we need to make sure that we have a basic React application set up. If you do not have a React application set up, please follow the instructions in the React documentation.
Once you have a basic React application set up, we can install React Router DOM by running the following command:
npm install react-router-dom
After React Router DOM has been installed, we can import it into our React application by adding the following line to the top of our App.js file:
import { BrowserRouter as Router, Route } from 'react-router-dom';
Now that we have imported React Router DOM into our application, we can start using it to create routes.
Creating Routes
In order to create a route, we need to use the <Route>
component. The <Route>
component takes two props:
- path: This is the path that the route will be accessible at.
- component: This is the React component that will be rendered when the route is accessed.
Let’s take a look at an example:
import React from 'react';
import { BrowserRouter as Router, Route } from 'react-router-dom';
function HomePage() {
return (
<div>
This is the home page.
</div>
);
}
function AboutPage() {
return (
<div>
This is the about page.
</div>
);
}
function App() {
return (
<Router>
<div>
<Route path="/" component={HomePage} />
<Route path="/about" component={AboutPage} />
</div>
</Router>
);
}
export default App;
JSXIn the above example, we have created two routes. The first route is for the home page, and the second route is for the about page. The home page route will be accessible at the root path ( / ), and the about page route will be accessible at the “/about” path.
When either of these routes is accessed, the corresponding React component will be rendered. In this case, the HomePage component will be rendered when the / route is accessed, and the AboutPage component will be rendered when the /about route is accessed.
Creating Linked Routes
In addition to creating routes, React Router DOM also provides a way to create links between routes. This is done with the <Link>
component. The <Link>
component takes two props:
- to: This is the path that the link will lead to.
- component: This is the React component that will be rendered as the link.
Let’s take a look at an example:
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function HomePage() {
return (
<div>
This is the home page.
<Link to="/about">About</Link>
</div>
);
}
function AboutPage() {
return (
<div>
This is the about page.
</div>
);
}
function App() {
return (
<Router>
<div>
<Route path="/" component={HomePage} />
<Route path="/about" component={AboutPage} />
</div>
</Router>
);
}
export default App;
JSXIn the above example, we have created a link on the home page that leads to the about page. When the About link is clicked, the about page route will be accessed, and the AboutPage component will be rendered.
Protecting React Router
React Router is vulnerable to a denial of service attack when used in conjunction with React Router DOM. The attack can be executed by a malicious user who is able to control the router’s history. The attack works by first loading a page that contains a React Router component. The component will then be used to load another page, which contains a React Router DOM component. The malicious user can then use the router’s history.push()
method to add an infinite number of entries to the router’s history, causing the router to become unresponsive.
To protect against this attack, you should ensure that your React Router and React Router DOM components are always updated to the latest version. You can also use the React Router DOM History.block()
method to prevent the router from adding new entries to the history.
Now, if you’re using any third party service like Auth0, then there are a lots of things to do. For that I recommend you to read their official blog if you’re using react-router v5. But if you’re using the v6 then I’d recommend you to read this blog “How to implement Auth0 protected route in react-router v6“.
What is the different between them?
Well the main problem difference is-
The object “Switch” was supported by react router-dom version 5, but it is replaced in react router-dom v6. Also in version 6 you can not use <ProtectedRoute>
.
So, simply you can not do this-
<Switch>
<Route path="/" exact component={Home} />
<ProtectedRoute path="/profile" component={Profile} />
</Switch>
JSXTo protect your routes you need to simply do this instead-
<Routes>
<Route path="/" exact element={<Home />} />
<Route path="/about" element={<About />} />
<Route element={<PrivateRoute/>}>
<Route path="/dashboard" element={<Dashboard/>} />
{/* Other Routes you want to protect */}
</Route>
</Routes>
JSXNow, as we’ve started the discussion of the differences, let’s see what are the updates react router v6 has comeup with.
What is new in React Router v6?
React Router v6 is the latest version of the popular open-source routing library for React applications. It introduces several new features and changes compared to its previous version, v5. Here are some of the notable updates in React Router v6:
- Dynamic Routing: React Router v6 now supports dynamic routing, allowing developers to define routes at runtime, instead of hardcoding them in the component.
- Hooks-based API: React Router v6 now uses a hooks-based API, which makes it easier for developers to manage and control the routing state in their React applications.
- Better Performance: React Router v6 has been optimized for better performance, making it faster and more efficient compared to its previous version.
- Conditional Routing: React Router v6 allows developers to define routes based on certain conditions, such as authentication status or device type.
- Nested Routes: React Router v6 supports nested routes, which allows developers to define child routes within a parent route.
- Server-side Rendering: React Router v6 supports server-side rendering, making it possible to pre-render the application on the server and improve the performance for users with slow internet connections.
- Smaller Bundle Size: React Router v6 has a smaller bundle size compared to its previous version, making it more suitable for applications with limited resources or low-bandwidth networks.
For more information about the v6, you can read the react router-dom documentation.
Now, let’s see some FAQs.
What is react router vs react router dom?
React Router and React Router DOM are both libraries for building routing and navigation in React applications, but they serve different purposes:
1. React Router: This is the core library that provides the routing logic and management. It provides an interface for defining the routes, handling navigation, and managing the routing state.
2. React Router DOM: This is the library that provides the components for rendering the routing configuration in a browser environment. It includes components such as Route
, Link
, Switch
, and Redirect
, which allow you to define the routes and navigation in your React application.
In other words, React Router provides the underlying logic for managing the routing state, while React Router DOM provides the components for rendering the routes in the browser.
When building a React application with routing capabilities, you typically use both libraries together. You would use the React Router core library to define the routes, handle navigation, and manage the routing state, and you would use the React Router DOM library to render the routing configuration in the browser.
If you got any new question, feel free to ask, and I’ll add it here.
Conclusion
In this guide, we have taken a look at how to use React Router DOM to create basic routes and links between routes. React Router DOM is a powerful routing package that enables developers to declaratively map routes to React components.