Follow Us:
How to Dockerize a React Native App?
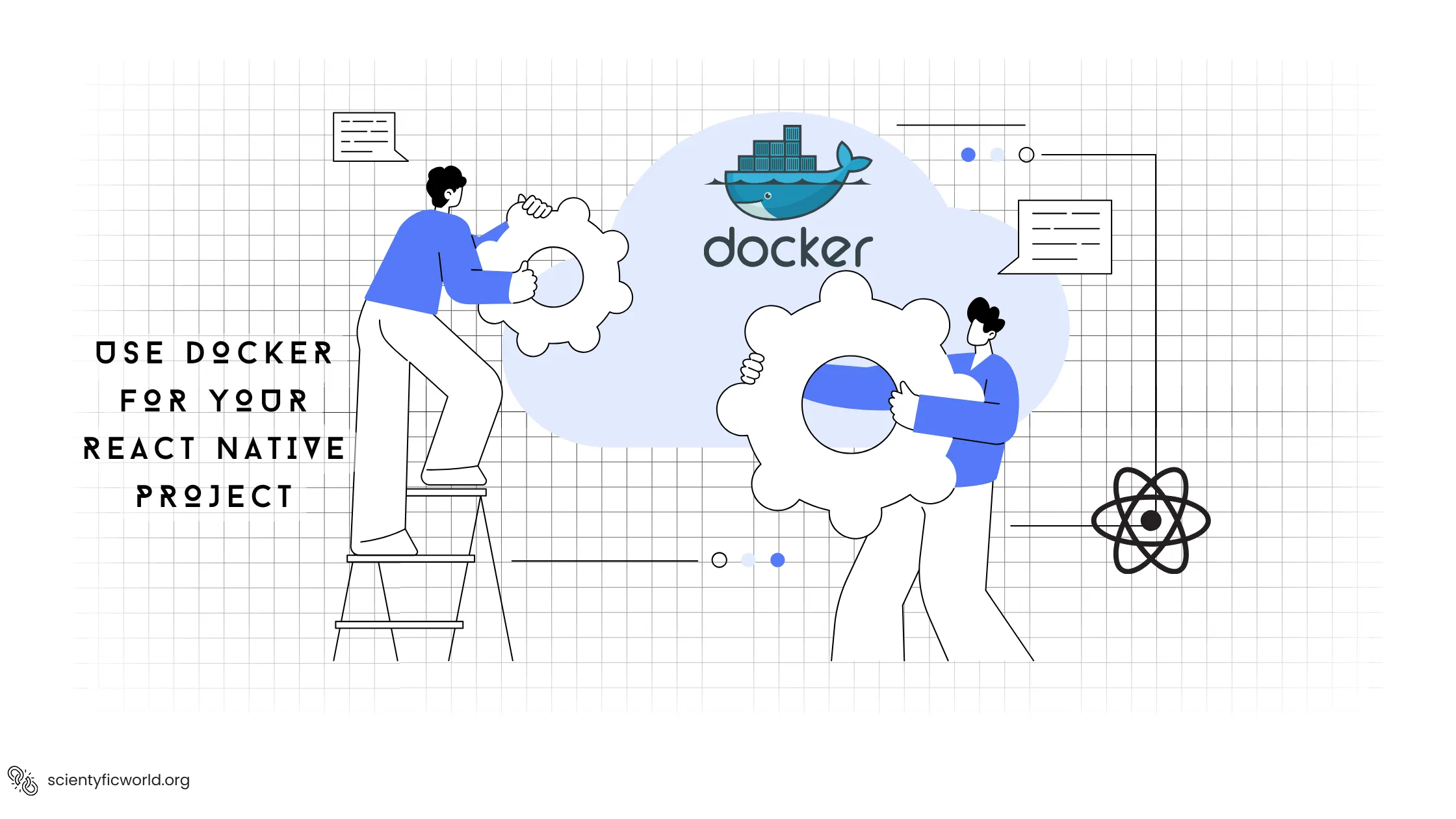
Dockerize a React Native application introduces a layer of consistency and efficiency across development, testing, and production environments. This guide aims to equip React Native developers with a structured approach to integrate Docker, enhancing project scalability and streamlining workflows. We’ll navigate through setting up your React Native project for Docker, optimizing the Dockerfile, running your app within a Docker container, and tackling advanced Docker functionalities relevant to React Native development. Tailored for developers at all levels, this guide ensures you leverage Docker to its full potential, addressing common challenges and providing troubleshooting insights. Join us as we demystify the process of dockerizing a React Native app, setting the stage for a more productive and controlled development environment.
Prerequisites
Before we dive into the process of dockerizing a React Native app, it’s essential to ensure you have the following prerequisites covered. This preparation will facilitate a smooth and efficient setup, allowing you to focus on the integration without interruptions:
- Docker Installation: You must have Docker installed on your machine. Docker is available for Windows, macOS, and Linux. If you haven’t installed Docker yet, please visit the official Docker website for installation instructions tailored to your operating system.
- React Native Development Environment: A basic React Native development environment should already be set up on your machine. This includes having Node.js installed, along with either the React Native CLI for native projects or Expo CLI for managed projects. If you need guidance on setting up your React Native environment, refer to the React Native environment setup documentation.
- Basic Understanding of Docker: Familiarity with Docker’s core concepts, such as containers, Dockerfiles, and Docker Compose, is crucial. This knowledge will help you grasp the dockerization process more effectively. If you’re new to Docker, consider going through the Docker Getting Started Guide.
- React Native Project: You should have an existing React Native project or be prepared to create a new one for this guide. This project will be used to demonstrate the dockerization process.
- Version Control: Although not strictly required, having your project under version control with Git is recommended. This practice is beneficial for managing changes and collaborating with others.
With these prerequisites in place, you’re well-prepared to start the journey of integrating Docker into your React Native app development workflow. This setup will not only enhance your development process but also align your project closer to industry standards for deployment and scalability.
What is Docker?
Docker is a powerful containerization platform that enables developers to package applications and their dependencies into containers. A container is a lightweight, standalone executable package that includes everything needed to run a piece of software, including the code, runtime, libraries, and system settings.
Below are key concepts essential to grasp when working with Docker:
- Containers vs Virtual Machines: Unlike virtual machines (VMs) that virtualize the hardware, containers virtualize the operating system. This makes containers more lightweight, as they share the host system’s kernel and require less overhead than running a full VM.
- Docker Images & Dockerfiles: Docker images are the blueprints of containers, defining what they should contain and how they should operate. Dockerfiles are text documents that contain all the commands a user could call on the command line to assemble an image. Using a Dockerfile, developers can build a Docker image which can then be used to create containers.
What does it mean by “Dockerize React Native App”?
“Dockerizing a React Native App” refers to the process of creating a Docker image that encapsulates a React Native application along with its dependencies, configurations, and environment settings. This allows the React Native app to be run consistently across different environments without worrying about compatibility issues or differences in the host system. By dockerizing a React Native app, developers can ensure portability, reproducibility, and easier deployment of their applications.
Why Use Docker for React Native?
Integrating Docker into React Native development addresses several common challenges while offering numerous benefits:
- Challenges of Development Environments: Development environments can vary significantly across machines, leading to the “it works on my machine” syndrome. Dependency variations and differing OS or tool versions can cause inconsistencies, hindering collaboration and productivity.
- Benefits of Docker for React Native Development:
- Consistent Development Environment Across Machines: Docker ensures that every member of the team works in an identical development environment, minimizing discrepancies between machines and operating systems. This uniformity simplifies development and debugging processes.
- Easier Collaboration and Onboarding of New Team Members: With Docker, setting up a development environment becomes as simple as running a few commands. This ease of setup accelerates the onboarding process for new team members and enhances collaboration within the team.
- Streamlined CI/CD Pipelines: Docker containers can be integrated into Continuous Integration/Continuous Deployment (CI/CD) pipelines, ensuring that builds are reproducible and that deployment environments match development environments closely. This alignment reduces deployment risks and facilitates a smoother release process.
- Potential for Isolated Testing Environments: Docker allows developers to create isolated environments for testing new features or updates. These isolated environments can mimic production settings closely without affecting the main development setup, enabling thorough and accurate testing.
By using Docker, React Native developers can mitigate environment-specific issues, enhance team collaboration, and streamline their development and deployment processes. The next sections will guide you through setting up your React Native project for Docker and exploring advanced Docker concepts to further optimize your workflow.
Setting Up Your Project
To successfully dockerize a React Native app, you’ll begin by ensuring your project is correctly set up and ready for Docker integration. This section covers the initial setup of a React Native project followed by the configuration of Docker for the application.
React Native Project Setup
If you don’t already have a React Native project, you’ll need to create one. Start by opening your terminal and running the command npx react-native init MyReactNativeApp
to initialize a new React Native project. This command creates a directory named MyReactNativeApp
with all the necessary files to get started with a React Native application.
Once the project setup is complete, navigate into your project directory using cd MyReactNativeApp
and run npx react-native run-android
or npx react-native run-ios
to ensure the project runs correctly on your emulator or device.
Setting Up Docker for the Application
After establishing your React Native project, the next step involves setting up Docker. This process includes creating a Dockerfile that specifies the environment for your application.
- Create a Dockerfile: In the root of your React Native project, create a file named
Dockerfile
. This file will define the Docker image for your application. - Define the Base Image: Start your Dockerfile by specifying a base image. For React Native, an image with Node.js is appropriate as it meets the runtime requirement. You can use the official Node image:
FROM node:latest
- Set Up the Working Directory: Specify a working directory in your Docker container. This directory will be the context for running project commands and where your project files will reside:
WORKDIR /app
- Copy Project Files: Copy your React Native project files into the Docker image. It’s important to note that you may want to exclude node_modules by using a
.dockerignore
file, similar to.gitignore
, to speed up the build process:COPY . .
- Install Dependencies: In the Dockerfile, add a command to install your project’s dependencies. Since React Native projects use npm or yarn, you can use:
RUN npm install
Or if you’re using yarn:RUN yarn install
- Exposing Ports: For development purposes, you might need to expose certain ports, especially if you’re running a server for your application or using hot reloading:
EXPOSE 8081
- Running Your App: Finally, define the command to run your app. This step can vary depending on your development setup. For example, you might start your React Native app for development:
CMD ["npx", "react-native", "start"]
With the Dockerfile in place, build the Docker image for your React Native project by running docker build -t myreactnativeapp .
in your project directory. This command creates an image named myreactnativeapp
based on the Dockerfile.
To run your React Native app inside a Docker container, use docker run -d -p 8081:8081 myreactnativeapp
, mapping the port to the one exposed in the Dockerfile.
This setup marks the initial steps toward integrating Docker into your React Native development process. It ensures your application is containerized, providing a consistent environment that mirrors production settings. In the following sections, we’ll dive deeper into optimizing this setup for development and deploying stages.
Dockerizing the React Native Environment
Having set up your React Native project and introduced Docker into your workflow, the next step is to delve deeper into dockerizing your development environment. This phase is crucial for ensuring your app runs smoothly within a Docker container, mirroring your production environment as closely as possible. Let’s explore how to optimize this setup for a seamless development experience.
Refining the Dockerfile for React Native Development
After initially setting up the Dockerfile, refining it further can enhance your React Native development experience. This step involves optimizing the environment specifically for the needs and challenges of React Native apps. Without repeating previously covered basics, let’s focus on advanced configurations and optimizations.
- Optimizing Layer Caching: Docker builds images in layers, allowing for efficient caching. By carefully ordering commands, you can take advantage of this feature to speed up rebuilds. For instance, copying the
package.json
andyarn.lock
orpackage-lock.json
before the rest of your files allow Docker to cache your node modules unless these files change.
COPY package.json yarn.lock ./
RUN yarn install
COPY . .
Zsh- Integrating Environment Variables: React Native apps often rely on environment variables for configuration. You can use the
ENV
instruction in your Dockerfile to set environment variables that your app can access at runtime.ENV API_URL=https://api.example.com
For dynamic environments, consider passing variables at runtime using Docker’s-e
flag when running your container. - Including Android SDK for Native Builds: If your workflow includes building Android APKs from within the Docker container, incorporating the Android SDK can be beneficial. This is a more advanced use case, requiring you to extend the Dockerfile to download and set up the Android SDK.
# Specify a base image with both Node.js and Java, or install Java in the current image
RUN apt-get update && apt-get install -y openjdk-8-jdk
# Download and install Android SDK
RUN wget -q <https://dl.google.com/android/repository/sdk-tools-linux-4333796.zip> -O android-sdk.zip && \\
unzip android-sdk.zip -d /usr/local/android-sdk && \\
rm android-sdk.zip
ENV ANDROID_HOME=/usr/local/android-sdk
ENV PATH=$PATH:$ANDROID_HOME/tools:$ANDROID_HOME/platform-tools
Zsh- Configuring Networking for Emulators: When running Android or iOS emulators outside Docker but serving the app from within Docker, network configuration becomes crucial. Ensure the Docker container and the emulator can communicate, which might involve configuring network bridges or using the host network.
# Example for a network configuration
EXPOSE 19000 19001
ZshUse Docker’s networking options (--network host
or custom bridges) to facilitate communication between the container and emulators running natively on your host system.
- Finalizing the Dockerfile: Ensure your Dockerfile ends with the command to start the React Native development server, as previously mentioned. This setup serves as the default action when the Docker container runs, keeping the development workflow straightforward and efficient.
Refining your Dockerfile with these considerations turns a basic Docker environment into a powerful tool for React Native development. By optimizing for caching, integrating essential tools and SDKs, and ensuring smooth networking, you create a robust, scalable, and efficient development environment that mirrors production conditions closely.
Building the Docker Image
With the Dockerfile optimized for React Native development, building your Docker image is the next step in encapsulating your development environment. This phase ensures that all the enhancements and configurations you’ve meticulously added to your Dockerfile are compiled into a usable Docker image. To refine this process, let’s focus on advanced strategies and tips for efficient image building, seamlessly integrating this step into your development workflow.
- Using BuildKit for Improved Performance: Docker’s BuildKit offers advanced features like efficient caching and parallel building, significantly improving build performance. Ensure BuildKit is enabled by setting the environment variable
DOCKER_BUILDKIT=1
before initiating the build process. This can be done by prepending the variable to your build command:DOCKER_BUILDKIT=1 docker build -t myreactnativeapp .
- Utilize Multi-Stage Builds: For more complex applications that require a build process before running (e.g., compiling TypeScript to JavaScript), consider using multi-stage builds in your Dockerfile. This approach allows you to separate the built environment from the runtime environment, reducing the final image size and keeping only the necessary artifacts:
# Build stage
FROM node:latest as builder
WORKDIR /app
COPY package.json yarn.lock ./
RUN yarn install
COPY . .
RUN yarn build
# Runtime stage
FROM node:alpine
WORKDIR /app
COPY --from=builder /app/build /app
EXPOSE 8081
CMD ["node", "index.js"]
Zsh- Tagging and Versioning: Properly tagging your Docker images can streamline your workflow, especially when working with multiple environments or versions. Use semantic versioning or environment-specific tags to distinguish between different builds:
docker build -t myreactnativeapp:1.0.0 .
- Automating the Build Process: Integrate the Docker build process into your automated workflows using scripts or continuous integration (CI) pipelines. This automation ensures that your Docker image is always up-to-date with your source code changes, facilitating continuous development and testing.
- Regularly Clean Up Unused Images: Over time, your system may accumulate a variety of Docker images, especially if you’re frequently building new versions. Regularly cleaning up unused images can free up significant disk space. Use the
docker image prune
command to remove unused images, keeping your development environment tidy.
By refining the Docker image-building process with these advanced strategies, you ensure an efficient, optimized containerization workflow for your React Native app. This streamlined approach not only enhances your development efficiency but also aligns with best practices for Docker usage in a professional development setting. With your Docker image now built and optimized, you’re well-prepared to run your React Native application within Docker, benefiting from a consistent, containerized development and testing environment.
Running the React Native App in a Docker Container
With your Docker image refined and built, the next pivotal step is to run your React Native app within a Docker container. This process brings your dockerization efforts to fruition, allowing you to see your app come to life in a containerized environment. The focus here is on executing this step effectively, ensuring a smooth transition for your app to operate within Docker.
- Starting the Container: Use the
docker run
command to create and start a container from your image. Specify any necessary flags to match your development setup, such as port forwarding to enable communication with the React Native Metro Bundler or any other services your app might use:docker run -d -p 8081:8081 myreactnativeapp
In this command,-d
runs the container in detached mode, allowing your terminal to be free for other commands, and-p 8081:8081
forwards the port from the container to your host, essential for accessing the Metro Bundler from outside the container. - Accessing the App: Once the container is running, your React Native app should be accessible in the manner you’ve configured it. If you’re running a web version via Expo or a similar service, you can access it through the forwarded port. For mobile versions, ensure your emulator or physical device can access the Docker-hosted service, adjusting network configurations if necessary.
- Live Reloading and Hot Refreshing: To take full advantage of React Native’s development features like live reloading and hot refreshing, ensure your Docker setup supports efficient file watching. This might involve configuring Docker volumes to mirror your local development files within the container, enabling changes to be reflected immediately:
docker run -d -p 8081:8081 -v $(pwd):/app myreactnativeapp
Here,-v $(pwd):/app
creates a volume that maps your project directory to the/app
directory in the container, facilitating live updates. - Debugging and Logging: Monitoring your app’s behavior is crucial during development. Use Docker’s logging capabilities to keep an eye on your app’s output. You can access logs of a running container with:
docker logs <container_id>
Replace<container_id>
with the ID of your container, obtainable by runningdocker ps
. - Iterative Development: As you make changes to your React Native app, you may need to rebuild your Docker image and restart the container to reflect those changes. Automating this process through scripts or integrating it into your development tools can greatly enhance productivity.
- Cleaning Up: To stop and remove your container when you’re done testing or developing for the day, use:
docker stop <container_id>
docker rm <container_id>
ZshKeeping your development environment clean avoids unnecessary resource consumption and potential conflicts with new containers.
By running your React Native app in a Docker container, you encapsulate your development environment, ensuring consistency across all team members’ setups and alignment with production environments. This containerized approach facilitates a more reliable and efficient development process, paving the way for seamless transitions between the development, testing, and production stages.
Advanced Docker Concepts for React Native
After mastering the basics of running a React Native app in a Docker container, diving into more advanced Docker concepts can further enhance your development workflow. These concepts address common challenges and open up new possibilities for efficiency and collaboration. Let’s explore some advanced Docker features that are particularly beneficial for React Native development.
Multi-Container Applications with Docker Compose
React Native apps often depend on backend services, databases, or other external services during development. Docker Compose simplifies the management of multi-container setups, allowing you to define and run multi-container Docker applications with ease.
- Docker Compose File: Create a
docker-compose.yml
file in your project root. This file describes your app’s services, networks, and volumes in a single YAML file, making it easy to start all components with a single command. - Service Configuration: Define services for your React Native app, backend API, database, and any other dependencies. Specify build contexts, environment variables, ports, and volumes for each service to ensure they interact correctly.
- Starting Services: Use
docker-compose up
to start your entire stack defined in thedocker-compose.yml
file. Docker Compose takes care of the networking between containers, making it straightforward to connect your React Native app to backend services.
Efficient Development with Docker Volumes
Docker volumes are crucial for creating an efficient development environment. They allow you to synchronize the code between your host and the container, enabling features like hot reloading and live debugging.
- Volume Configuration: In your Dockerfile or
docker-compose.yml
, define volumes that map your React Native project directory to the corresponding directory inside the container. This setup ensures that changes made on your host are immediately available inside the container. - Live Reloading: With volumes set up, changes to your React Native code trigger the hot reloading mechanism, instantly refreshing your app with the new changes without rebuilding the container.
Networking for Emulator or Device Testing
Connecting your Dockerized React Native app to an emulator or physical device for testing can require additional networking configurations.
- Host Network: For the simplest setup, especially when testing on emulators running on the same host machine, you can use the host’s network directly by specifying
network_mode: "host"
in yourdocker-compose.yml
. This method makes the container’s network stack identical to the host, simplifying connectivity. - Custom Bridges: For more complex scenarios or specific networking needs, you can define custom network bridges in Docker. This approach allows for fine-grained control over container networking, enabling specific routing and port forwarding rules as needed.
Automated Testing Environments
Docker can be leveraged to create isolated environments for automated testing, ensuring that tests run in a consistent, controlled setting.
- Dockerized Test Suites: Containerize your test suites alongside your application. Running tests in containers guarantees that they execute in the same environment every time, reducing “works on my machine” issues.
- CI/CD Integration: Integrate Dockerized tests into your CI/CD pipelines. Most CI/CD tools support Docker, allowing you to run tests in containers as part of your deployment process, ensuring code quality and reliability.
By embracing these advanced Docker concepts, you can address common development challenges, improve productivity, and ensure consistency across development, testing, and production environments. Docker’s flexibility and powerful features offer a robust solution for developing and deploying complex React Native applications.
Common Docker Issues and Troubleshooting:
Even with a well-set-up Docker environment for React Native development, developers might encounter specific issues. Identifying these common problems and applying effective troubleshooting strategies can save time and prevent frustration. Here’s a focused guide on navigating some typical challenges and their solutions.
- Networking Challenges with Emulators or Devices:
Issue: Your React Native app running inside a Docker container may not connect to backend services or be inaccessible from an emulator or physical device.
Solution: Ensure the Docker container’s network is correctly configured to allow connections from your emulator or device. Using Docker’s host network mode can simplify connectivity for emulators running on the same machine. For devices, ensure the device is on the same network and proper port forwarding is set up in Docker. - Hot Reloading Not Working:
Issue: Changes made to your React Native code do not trigger hot reloading or live updates in the app.
Solution: This issue often arises due to misconfigured Docker volumes. Ensure yourdocker run
command ordocker-compose.yml
file correctly maps your project directory to the container’s working directory. Additionally, verify that your React Native development server inside Docker is configured to watch for file changes. - Performance Issues on Non-Linux Hosts:
Issue: Developers using Docker Desktop on macOS or Windows might experience slower performance, particularly with file system operations.
Solution: Utilize Docker’s caching mechanisms or consider increasing Docker Desktop’s allocated resources (CPU and memory) through its settings. Additionally, exploring options like Docker’s:delegated
or:cached
volume flags can improve performance for mounted volumes. - Container Fails to Start:
Issue: The Docker container exits immediately after starting, preventing the React Native app from running.
Solution: This often indicates an issue with the command used to start the app within the Docker container. Check the Docker container logs usingdocker logs <container_id>
to identify any error messages. Ensure the Dockerfile’s CMD instruction correctly starts your React Native development server or the intended process. - Dependencies or Environment Mismatch:
Issue: The app behaves differently inside the Docker container compared to the host environment, possibly due to differences in dependencies or environment variables.
Solution: Rebuild the Docker image after any dependency changes to ensure the container has the latest versions. Use environment variables in Docker (defined in Dockerfile ordocker-compose.yml
) to match your development and production environments as closely as possible.
Debugging Tips
- Use Docker Logs: Docker logs are invaluable for understanding what’s happening inside your container. If your application or server fails to start, the logs can provide insight into what went wrong.
- Interactive Mode: Running the container in interactive mode with a shell (using
docker run -it <image> /bin/sh
) allows you to explore the container’s file system and run commands manually, which can be useful for debugging. - Simplify and Isolate: When facing complex issues, simplify your Docker setup to isolate the problem. This might involve creating a minimal Dockerfile that only includes the core components necessary to run your app.
By addressing these common issues with informed troubleshooting strategies, developers can maintain a smooth and efficient workflow when dockerizing React Native applications, ensuring minimal disruption to their development process.
Conclusion
Successfully dockerizing a React Native application marks a significant enhancement in your development workflow, offering a consistent, scalable, and isolated environment that mirrors production settings. Throughout this guide, we’ve navigated the intricacies of setting up and refining a Docker environment tailored for React Native, from initial Dockerfile creation to running your app in a Docker container and exploring advanced Docker concepts to address multifaceted development scenarios.
Addressing common issues and applying troubleshooting strategies further ensures a resilient setup, enabling developers to overcome typical challenges encountered during containerization. The insights provided aim to equip you with a robust foundation for integrating Docker into your React Native projects, enhancing collaboration, and streamlining the development to-deployment pipeline.
As you continue to explore and integrate Docker with React Native, remember that the containerization journey doesn’t end here. Continuously evolving your Docker practices, keeping abreast with the latest in Docker and React Native developments, and experimenting with new tools and techniques will drive further efficiencies and innovations in your projects.
In conclusion, the integration of Docker into React Native development encapsulates the spirit of modern application development—leveraging powerful tools to create more reliable, consistent, and scalable applications. Embrace these practices to not only improve your development process but also to foster a culture of technical excellence within your teams.
FAQs:
How do you handle native dependencies when Dockerizing a React Native app?
When Dockerizing a React Native app, managing native dependencies can be challenging due to differences in host and container environments. You may need to set up multi-stage builds, use platform-specific Docker images, or manually configure native dependencies within the Dockerfile.
What are the best practices for optimizing Docker builds for React Native apps?
Optimizing Docker builds for React Native apps involves minimizing image size, leveraging caching mechanisms, utilizing multi-stage builds, and carefully structuring the Dockerfile to ensure efficient layer caching and faster build times.
How can you securely manage sensitive information like API keys in a Dockerized React Native app?
Securing sensitive information such as API keys in a Dockerized React Native app requires using environment variables, Docker secrets, or external configuration management tools. It’s crucial to follow best practices for handling secrets in Docker containers to prevent exposure of sensitive data.
What strategies can be employed to streamline the deployment of Dockerized React Native apps to production environments?
Streamlining the deployment of Dockerized React Native apps to production involves setting up CI/CD pipelines, automating the build and deployment process, utilizing container orchestration tools like Kubernetes, and implementing blue-green deployments or canary releases for seamless updates.
How do you troubleshoot common issues when Dockerizing a React Native app?
Troubleshooting Dockerized React Native apps may involve debugging container networking, resolving compatibility issues between React Native and Docker, troubleshooting performance bottlenecks related to container resource allocation, and addressing issues with volume mounting or file permissions within the container. Utilizing logging, monitoring tools, and Docker-specific debugging techniques can help diagnose and resolve common issues effectively.